How to Implement Virtual Template Methods in C ?
C Virtual Template Method
The scenario presented involves an abstract class with virtual template methods, setData and getData, intended to dynamically set and retrieve data of various types based on a provided identifier. However, as the question acknowledges, such a construct is not directly supported in C .
To address this limitation, there are two primary approaches:
Remove Static Polymorphism
One option is to remove static polymorphism (templates) and introduce an intermediate storage mechanism. The AbstractComputation class could define a non-templated ValueStore, responsible for storing and retrieving data based on identifiers:
<code class="cpp">class ValueStore { public: template <typename T> void setData(std::string const &id, T value) {...} template <typename T> T getData(std::string const &id) {...} }; class AbstractComputation { public: template <typename T> void setData(std::string const &id, T value) { m_store.setData(id, value); } template <typename T> T getData(std::string const &id) const { return m_store.getData<T>(id); } private: ValueStore m_store; };</code>
Deriving classes can then directly access the ValueStore without the need for runtime polymorphism.
Remove Runtime Polymorphism
Alternatively, runtime polymorphism can be retained while removing static polymorphism. This involves performing type erasure on the base class:
<code class="cpp">class AbstractComputation { public: template <typename T> void setData(std::string const &id, T value) { setDataImpl(id, boost::any(value)); } template <typename T> T getData(std::string const &id) const { boost::any res = getDataImpl(id); return boost::any_cast<T>(res); } protected: virtual void setDataImpl(std::string const &id, boost::any const &value) = 0; virtual boost::any getDataImpl(std::string const &id) const = 0; };</code>
boost::any is a type that can store values of any type and allows for safe retrieval of the data. Type erasure is performed by passing boost::any objects as arguments to the virtual methods, which must be implemented in the derived classes.
The above is the detailed content of How to Implement Virtual Template Methods in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


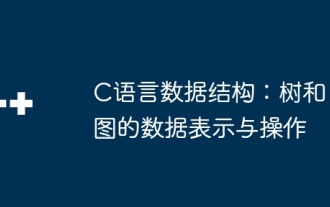
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
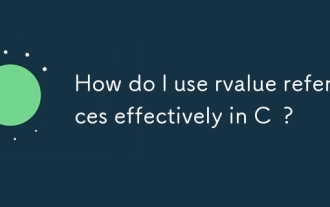
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
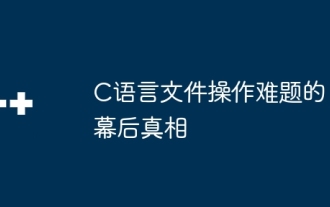
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
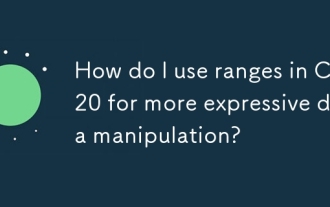
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
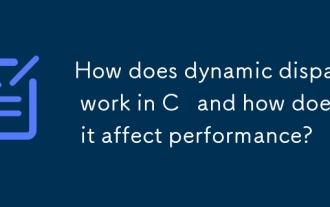
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
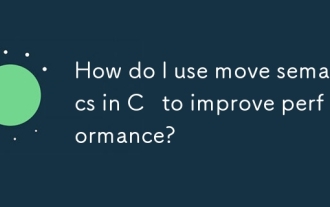
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
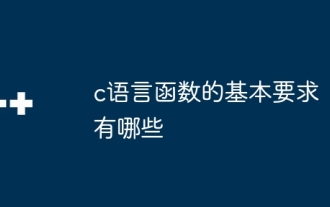
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
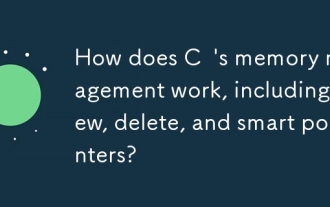
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
