How Can You Halt a Go For Loop Externally?
Halting a For Loop's Execution Externally in Go
In Go, you may encounter scenarios where you need to terminate the execution of a for loop from outside its scope. For instance, you might have a long-running loop that needs to be interrupted when a specific condition is met.
To achieve this, you can leverage a signaling channel. Here's how it works:
Creating a Signaling Channel
<code class="go">quit := make(chan struct{}{})</code>
This line creates a channel with an empty struct as its value type. It will be used to signal the loop to stop.
Closing the Channel
Within the goroutine that is running the loop, you can use the close function to close the signaling channel when the desired condition is met:
<code class="go">if count > 5 { close(quit) wg.Done() return }</code>
Reading from the Closed Channel
When the quit channel is closed, reading from it returns immediately with a zero value. This behavior is exploited in the outer loop. By default, reading from a closed channel blocks indefinitely. However, this can be circumvented using a select statement:
<code class="go">myLoop: for { select { case <-quit: break myLoop default: fmt.Println("iteration", i) i++ } }</code>
In this select statement, the default case continues the loop iteration as expected. When the quit channel is closed, the first case is satisfied, causing the loop to break immediately.
By using a signaling channel, you can effectively stop the execution of a for loop from an external function or goroutine when necessary, ensuring greater flexibility and control over your code execution.
The above is the detailed content of How Can You Halt a Go For Loop Externally?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










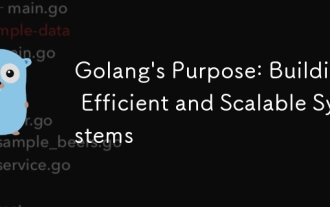
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
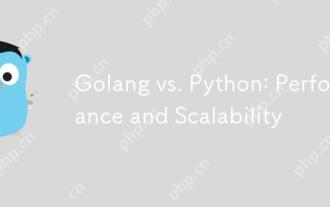
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
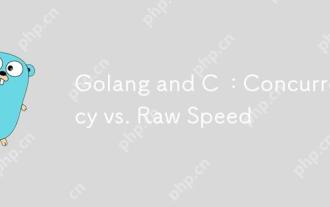
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
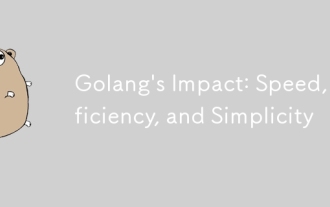
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
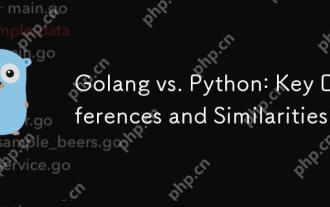
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
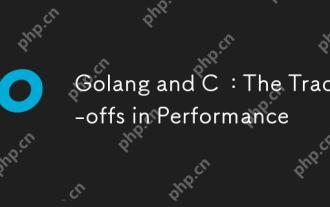
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
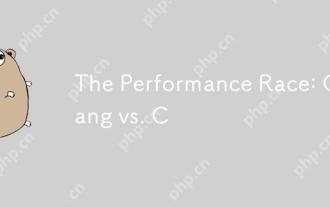
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
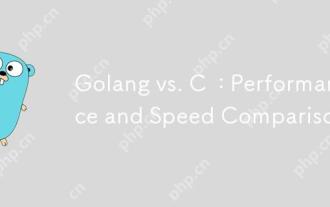
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
