Understanding Time Complexity in Python Functions
Understanding the time complexity of functions is crucial for writing efficient code. Time complexity provides a way to analyze how the runtime of an algorithm increases as the size of the input data grows. In this article, we will explore the time complexity of various built-in Python functions and common data structures, helping developers make informed decisions when writing their code.
What is Time Complexity?
Time complexity is a computational concept that describes the amount of time an algorithm takes to complete as a function of the length of the input. It is usually expressed using Big O notation, which classifies algorithms according to their worst-case or upper bound performance. Common time complexities include:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n²): Quadratic time
- O(2^n): Exponential time
Understanding these complexities helps developers choose the right algorithms and data structures for their applications.
Time Complexity of Built-in Python Functions
1. List Operations
-
Accessing an Element: list[index] → O(1)
- Accessing an element by index in a list is a constant time operation.
-
Appending an Element: list.append(value) → O(1)
- Adding an element to the end of a list is generally a constant time operation, although it can occasionally be O(n) when the list needs to be resized.
-
Inserting an Element: list.insert(index, value) → O(n)
- Inserting an element at a specific index requires shifting elements, resulting in linear time complexity.
-
Removing an Element: list.remove(value) → O(n)
- Removing an element (by value) requires searching for the element first, which takes linear time.
-
Sorting a List: list.sort() → O(n log n)
- Python’s built-in sorting algorithm (Timsort) has a time complexity of O(n log n) in the average and worst cases.
2. Dictionary Operations
-
Accessing a Value: dict[key] → O(1)
- Retrieving a value by key in a dictionary is a constant time operation due to the underlying hash table implementation.
-
Inserting a Key-Value Pair: dict[key] = value → O(1)
- Adding a new key-value pair is also a constant time operation.
-
Removing a Key-Value Pair: del dict[key] → O(1)
- Deleting a key-value pair is performed in constant time.
-
Checking Membership: key in dict → O(1)
- Checking if a key exists in a dictionary is a constant time operation.
3. Set Operations
-
Adding an Element: set.add(value) → O(1)
- Adding an element to a set is a constant time operation.
-
Checking Membership: value in set → O(1)
- Checking if an element is in a set is also a constant time operation.
-
Removing an Element: set.remove(value) → O(1)
- Removing an element from a set is performed in constant time.
4. String Operations
-
Accessing a Character: string[index] → O(1)
- Accessing a character in a string by index is a constant time operation.
-
Concatenation: string1 string2 → O(n)
- Concatenating two strings takes linear time, as a new string must be created.
-
Searching for a Substring: string.find(substring) → O(n*m)
- Searching for a substring in a string can take linear time in the worst case, where n is the length of the string and m is the length of the substring.
5. Other Common Functions
-
Finding Length: len(object) → O(1)
- Finding the length of a list, dictionary, or set is a constant time operation.
-
List Comprehensions: [expression for item in iterable] → O(n)
- The time complexity of list comprehensions is linear, as they iterate through the entire iterable.
Conclusion
By analyzing the performance of built-in functions and data structures, developers can make informed decisions that lead to better application performance. Always consider the size of your input data and the operations you need to perform when choosing the right data structures and
The above is the detailed content of Understanding Time Complexity in Python Functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










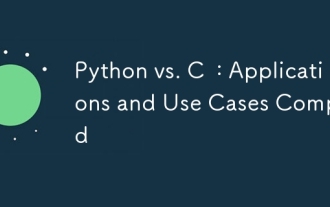
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
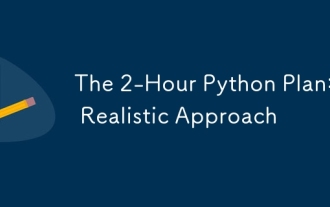
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
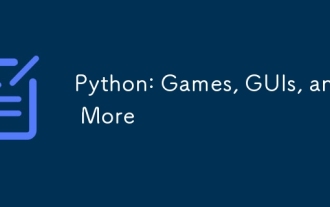
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
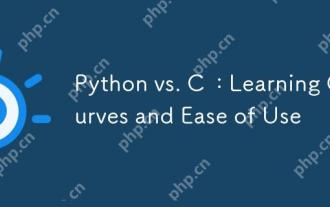
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
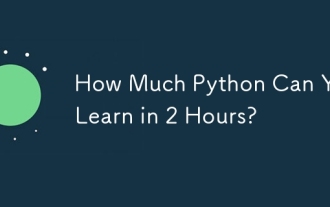
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
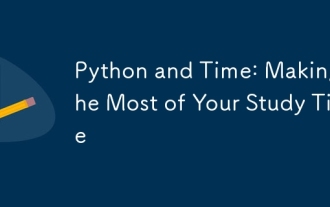
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
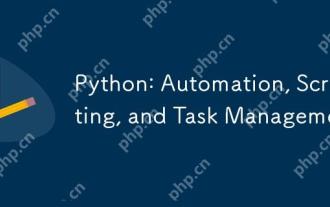
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
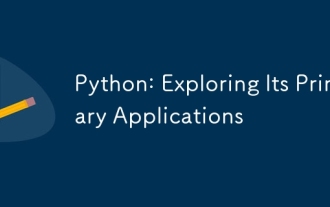
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
