How to Test Go Applications That Read from Stdin?
Testing Go Applications with Stdin Input
In Go, testing applications that read from stdin can be challenging. Consider a simple application that echoes stdin input to stdout. While it may seem straightforward, writing a test case that verifies the output can pose difficulties.
Failed Attempt
An initial approach would be to simulate stdin and stdout using pipes and manually write to the stdin pipe. However, this may lead to race conditions and unexpected failures.
Solution: Extract Logic and Test Independent Function
Instead of performing all operations in the main function using stdin and stdout, create a separate function that accepts io.Reader and io.Writer as parameters. This approach allows the main function to call the function while the test function directly tests it.
Refactored Code
<code class="go">package main import ( "bufio" "fmt" "io" ) // Echo takes an io.Reader and an io.Writer and echoes input to output. func Echo(r io.Reader, w io.Writer) { reader := bufio.NewReader(r) for { fmt.Print("> ") bytes, _, _ := reader.ReadLine() if bytes == nil { break } fmt.Fprintln(w, string(bytes)) } } func main() { Echo(os.Stdin, os.Stdout) }</code>
Updated Test Case
<code class="go">package main import ( "bufio" "bytes" "io" "os" "testing" ) func TestEcho(t *testing.T) { input := "abc\n" reader := bytes.NewBufferString(input) writer := &bytes.Buffer{} Echo(reader, writer) actual := writer.String() if actual != input { t.Errorf("Wanted: %v, Got: %v", input, actual) } }</code>
This test case simulates the main function by calling the Echo function directly with a buffer for stdin input and a buffer for capturing the output. The captured output is then compared to the expected input, ensuring that the function correctly echoes the input.
The above is the detailed content of How to Test Go Applications That Read from Stdin?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


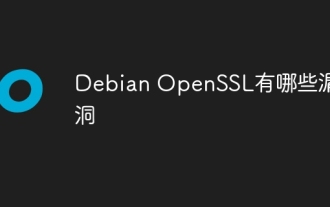
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
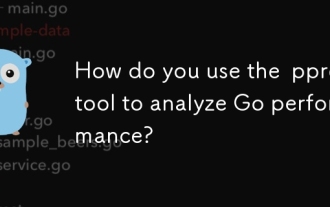
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
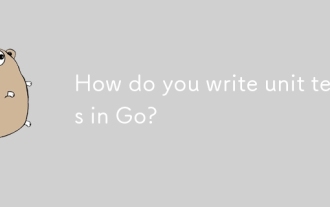
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
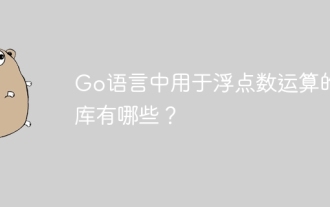
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
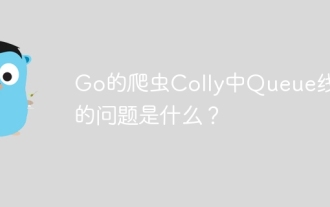
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
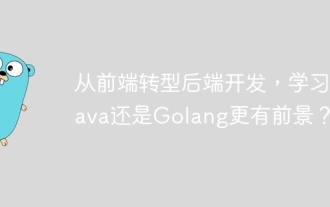
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
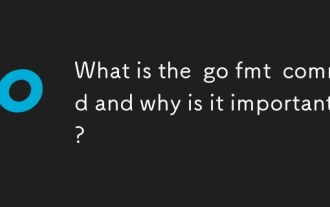
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
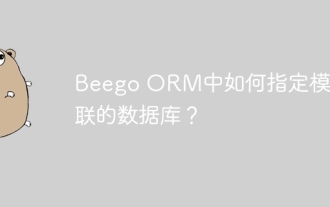
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
