


Here are a few title options based on the provided text, focusing on the \'question\' aspect: Option 1 (More Direct): * How do I run Cucumber feature files in parallel using the `cucumber-
Executing Cucumber Feature Files in Parallel
To execute Cucumber feature files in parallel, you can leverage a plugin called cucumber-jvm-parallel-plugin. Here's how to use it:
-
Add the Plugin to Your POM:
<code class="xml"><dependency> <groupId>com.github.temyers</groupId> <artifactId>cucumber-jvm-parallel-plugin</artifactId> <version>2.1.0</version> </dependency></code>
Copy after login -
Configure the Plugin in Your Build:
<code class="xml"><plugin> <groupId>com.github.temyers</groupId> <artifactId>cucumber-jvm-parallel-plugin</artifactId> <version>2.1.0</version> <executions> <execution> <id>generateRunners</id> <phase>generate-test-sources</phase> <goals> <goal>generateRunners</goal> </goals> <configuration> <!-- Package names for glue code --> <glue>com.example.cucumber</glue> <!-- Output directory for generated runner classes --> <outputDirectory>${project.build.directory}/generated-test-sources/cucumber</outputDirectory> <!-- Features directory --> <featuresDirectory>src/test/resources/features/</featuresDirectory> <!-- Output directory for Cucumber reports --> <cucumberOutputDir>target/cucumber-parallel</cucumberOutputDir> <!-- Output format --> <format>json</format> <!-- Strict property --> <strict>true</strict> <!-- Monochrome property --> <monochrome>true</monochrome> <!-- Tags to run --> <tags></tags> <!-- Filter features by tags --> <filterFeaturesByTags>false</filterFeaturesByTags> <!-- Use TestNG runners --> <useTestNG>false</useTestNG> <!-- Naming scheme --> <namingScheme>simple</namingScheme> <!-- Naming pattern --> <namingPattern>Parallel{c}IT</namingPattern> <!-- Parallel scheme --> <parallelScheme>SCENARIO</parallelScheme> </configuration> </execution> </executions> </plugin></code>
Copy after login -
Configure Surefire Plugin to Invoke TestNG Runners:
<code class="xml"><plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.19</version> <configuration> <forkCount>5</forkCount> <reuseForks>true</reuseForks> <includes> <include>**/*IT.class</include> </includes> </configuration> </plugin></code>
Copy after login -
Share the WebDriver Instance:
In order to avoid having different thread instances of the WebDriver, you must implement a shared WebDriver class that prevents the driver.quit() method from being called:<code class="java">import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.support.events.EventFiringWebDriver; public class SharedDriver extends EventFiringWebDriver { private static WebDriver REAL_DRIVER = null; public SharedDriver() { super(CreateDriver()); } public static WebDriver CreateDriver() { WebDriver webDriver; if (REAL_DRIVER == null) { webDriver = new FirefoxDriver(); setWebDriver(webDriver); } return webDriver; } public static void setWebDriver(WebDriver webDriver) { REAL_DRIVER = webDriver; } public static WebDriver getWebDriver() { return REAL_DRIVER; } @Override public void close() { if (Thread.currentThread() != CLOSE_THREAD) { throw new UnsupportedOperationException("You shouldn't close this WebDriver. It's shared and will close when the JVM exits."); } super.close(); } }</code>
Copy after login -
Configure Hub to Support Parallel Execution:
If you plan on running more than 50 threads, you need to increase the -DPOOL_MAX value for the Hub.java -jar selenium-server-standalone-<version>.jar -role hub -DPOOL_MAX=512
Copy after login -
Execute the Feature Files:
Run your Cucumber tests in parallel using the command:mvn test
Copy after login
The above is the detailed content of Here are a few title options based on the provided text, focusing on the \'question\' aspect: Option 1 (More Direct): * How do I run Cucumber feature files in parallel using the `cucumber-. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










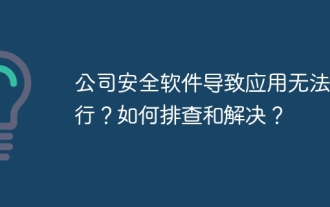
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
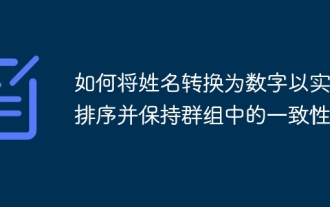
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
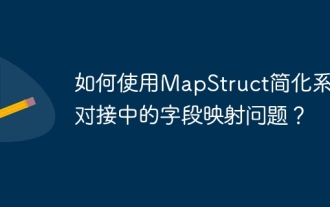
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
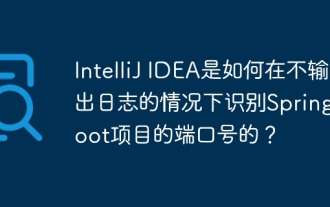
Start Spring using IntelliJIDEAUltimate version...
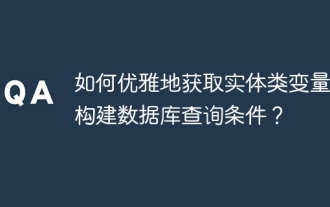
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
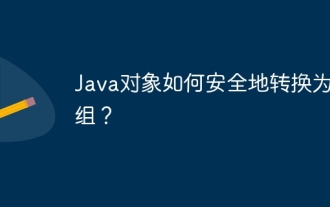
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
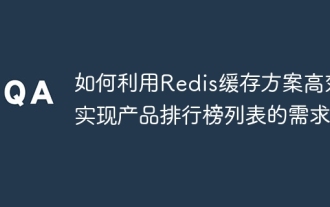
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
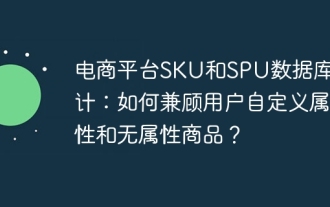
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
