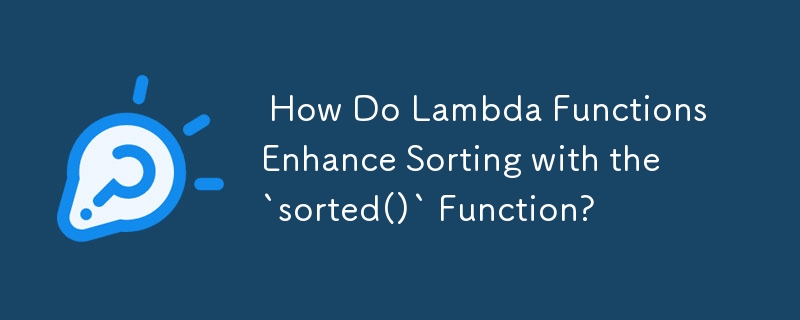
Why Use Lambda for Sorted Key Arguments?
The sorted() function requires specifying a key argument to sort elements in a particular way. Lambda expressions, despite their arbitrary appearance, serve a specific purpose in this context.
Intuition Behind Lambda
- Lambda functions are typically used when you need a single-line function without defining a separate function.
- They follow the syntax: lambda input_variable(s): expression
How Lambda Works for Sorted
- Lambda functions in sorted() provide instructions on extracting values from list elements that will guide the sorting process.
- The key argument says: "As we iterate through the list, use this function to transform each element into a value that will be used to sort."
Example
Consider the list [3, 6, 3, 2, 4, 8, 23] and the following lambda function:
lambda x: x % 2 == 0
- This lambda checks if each element in the list is even (x % 2 == 0).
- Sorted transforms the list based on this lambda function, resulting in a sequence of 1s (even numbers) and 0s (odd numbers): [0, 1, 0, 1, 1, 1, 0]
- The final sorted list is: [3, 3, 23, 6, 2, 4, 8]
Additional Points
- Even though the transformed list contains boolean values, the original order of the elements is retained within the sublists.
- The sorted() function performs sorting only once, resulting in even numbers still being unsorted.
- Lambda functions can extract values from complex elements like tuples, which aids in sorting lists containing nested structures.
Why variable: variable[0]
The syntax key=lambda variable: variable[0] is used when you want to sort a list of tuples based on the first element of each tuple. Here, variable represents each tuple, and variable[0] represents the first element within that tuple.
The above is the detailed content of How Do Lambda Functions Enhance Sorting with the `sorted()` Function?. For more information, please follow other related articles on the PHP Chinese website!