


How to Unmarshal a Comma-Separated String into a Slice in Go Using Custom Unmarshaling?
Custom Unmarshal with String Split in Go
When unmarshalling JSON into Go structs, the default behavior is to convert JSON values to the corresponding struct fields. However, there are scenarios where you may need to perform custom transformations during the unmarshalling process.
Consider a JSON object with "subjects" represented as a comma-separated string. To unmarshal this into a Go struct with "subjects" as a slice of strings, you need to split the string during unmarshalling.
One approach is to implement a custom unmarshaller for the "subjects" field using the json.Unmarshaler interface. Here's how you can achieve this:
type SubjectSlice []string // UnmarshalJSON implements custom unmarshalling for SubjectSlice. func (s *SubjectSlice) UnmarshalJSON(data []byte) error { var subjects string err := json.Unmarshal(data, &subjects) if err != nil { return err } *s = strings.Split(subjects, "-") return nil }
In your struct definition, use the custom slice type for the "subjects" field:
type Student struct { StudentNumber int Name string Subjects SubjectSlice }
When you unmarshal the JSON using this custom unmarshaller, the "subjects" field will be automatically split into a slice of strings.
For example, consider the following JSON:
{"student_number":1234567, "name":"John Doe", "subjects":"Chemistry-Maths-History-Geography"}
Unmarshaling it into the Student struct with the custom unmarshaller would result in:
s := Student{ StudentNumber: 1234567, Name: "John Doe", Subjects: []string{"Chemistry", "Maths", "History", "Geography"}, }
By implementing custom unmarshallers, you can handle complex data transformations during unmarshalling, making it a powerful tool for working with custom data structures in JSON.
The above is the detailed content of How to Unmarshal a Comma-Separated String into a Slice in Go Using Custom Unmarshaling?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










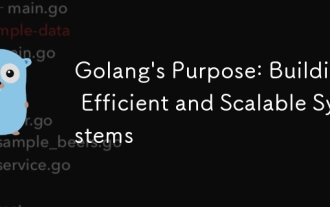
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
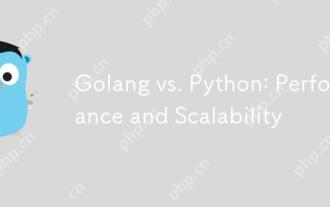
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
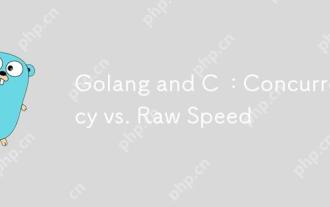
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
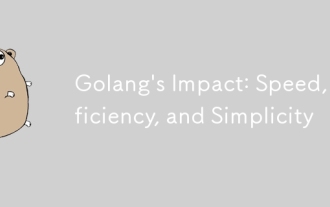
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
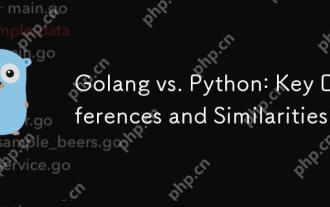
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
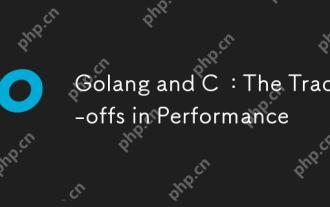
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
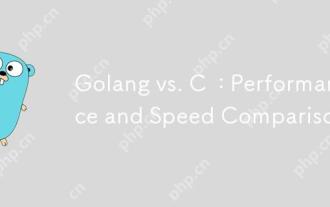
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
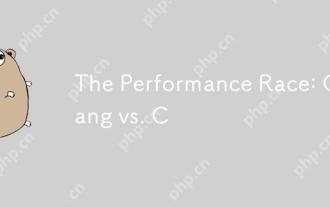
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
