


How to Unmarshal JSON with Arbitrary Key/Value Pairs into a Struct in Go?
Unmarshal JSON with Arbitrary Key/Value Pairs to Struct
Many similar questions have been asked regarding unmarshalling JSON with unknown/arbitrary key/value pairs into a struct. However, none of the solutions found provided a simple and elegant method to achieve this.
Problem Statement
We have a JSON string containing known fields (always present) and an unknown number of unknown/arbitrary fields. Example:
<code class="json">{"known1": "foo", "known2": "bar", "unknown1": "car", "unknown2": 1}</code>
In this example, known1 and known2 are known fields, while unknown1 and unknown2 are arbitrary fields. The unknown fields can have any name (key) and value (string, bool, float64, or int).
Our goal is to find an idiomatic way to parse such a JSON message into a struct.
Proposed Solution
We can define the following struct:
<code class="go">type Message struct { Known1 string `json:"known1"` Known2 string `json:"known2"` Unknowns []map[string]interface{} }</code>
With this struct, the sample JSON message should yield the following result:
{Known1:foo Known2:bar Unknowns:[map[unknown1:car] map[unknown2:1]]}
Alternative Solution
Another option is to unmarshal the JSON into a map[string]interface{}:
<code class="go">import ( "encoding/json" "fmt" ) func main() { jsonMsg := `{"known1": "foo", "known2": "bar", "unknown1": "car", "unknown2": 1}` var msg map[string]interface{} fmt.Println(json.Unmarshal([]byte(jsonMsg), &msg)) fmt.Printf("%+v", msg) }</code>
This will produce:
<nil> map[known1:foo known2:bar unknown1:car unknown2:1]
The advantage of this approach is that we can iterate over the keys and values and perform necessary type assertions to handle the data. We may or may not populate a struct with the data depending on our needs.
The above is the detailed content of How to Unmarshal JSON with Arbitrary Key/Value Pairs into a Struct in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










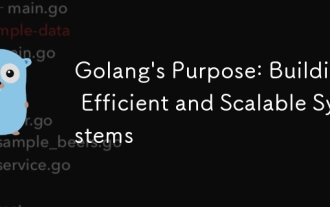
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
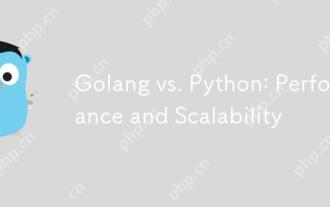
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
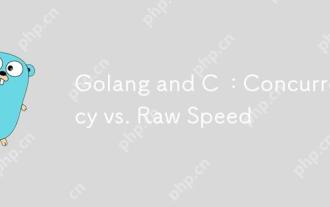
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
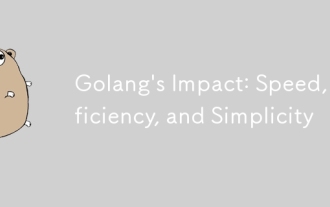
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
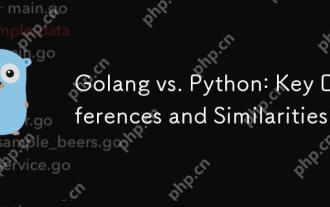
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
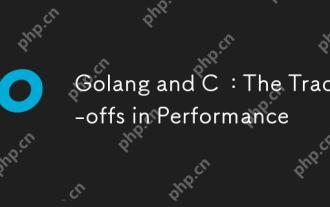
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
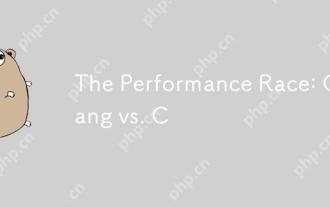
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
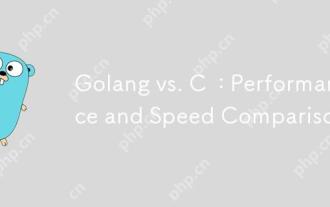
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
