


Why is my PHP WebSocket server failing to establish a connection and showing the error \'Firefox can\'t establish a connection to the server at ws://localhost:12345/\'?
How to Create WebSocket Server in PHP
Problem
Creating a WebSocket server in PHP can be challenging, especially when using outdated or buggy libraries. Despite numerous attempts, the code provided in the question fails to establish a connection, resulting in the error: "Firefox can't establish a connection to the server at ws://localhost:12345/."
Solution
To overcome this issue, consider the following steps:
- Understand WebSocket Protocol: Read the WebSocket draft to grasp the protocol's inner workings. Refer to it regularly throughout the development process.
- Create a Handshake Procedure: Implement a proper handshake procedure based on the instructions in the WebSocket draft.
- Handle WebSocket Message Masking: Data sent and received over WebSocket is typically masked. Implement encoding and decoding logic to account for this.
- Handle Connection Closing: Add logic to handle the closing of WebSocket connections.
- Use a Reliable WebSocket Library: Consider using a well-maintained WebSocket library like Ratchet or Symfony Websocket.
- Test and Troubleshoot: Thoroughly test the server and debug any errors or connection issues.
Code Example
The following PHP code provides a simple example of a WebSocket server implementation using Ratchet:
<code class="php">use Ratchet\MessageComponentInterface; use Ratchet\ConnectionInterface; class MyWebSocketHandler implements MessageComponentInterface { private $clients; public function __construct() { $this->clients = new SplObjectStorage; } public function onOpen(ConnectionInterface $conn) { // Send a welcome message to the client $conn->send('Welcome to the WebSocket server!'); // Add the client to the collection $this->clients->attach($conn); } public function onMessage(ConnectionInterface $from, $msg) { // Send the message to all connected clients foreach ($this->clients as $client) { $client->send($msg); } } public function onClose(ConnectionInterface $conn) { // Remove the client from the collection $this->clients->detach($conn); } public function onError(ConnectionInterface $conn, \Exception $e) { // Handle errors $conn->close(); } }</code>
Conclusion
Creating a WebSocket server in PHP requires in-depth understanding of the WebSocket protocol and proper implementation of its features. By following the presented steps and leveraging reliable libraries, it is possible to establish a robust WebSocket server that facilitates real-time communication.
The above is the detailed content of Why is my PHP WebSocket server failing to establish a connection and showing the error \'Firefox can\'t establish a connection to the server at ws://localhost:12345/\'?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
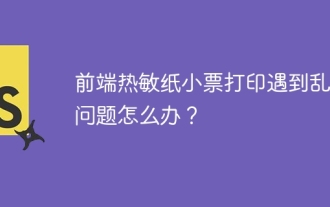
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
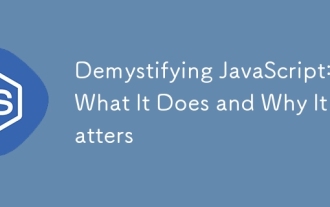
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
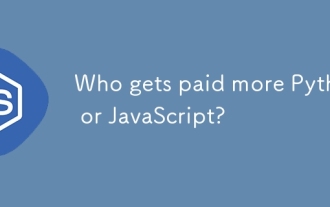
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
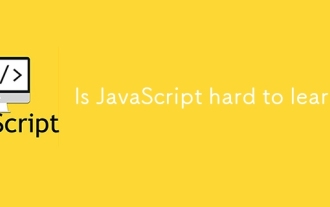
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
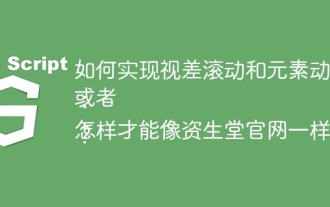
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
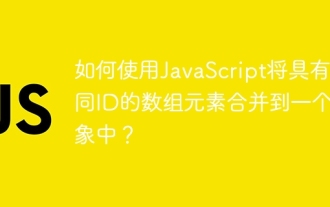
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
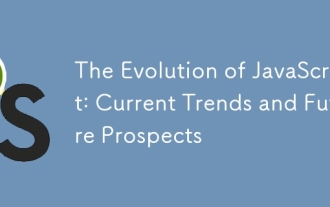
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
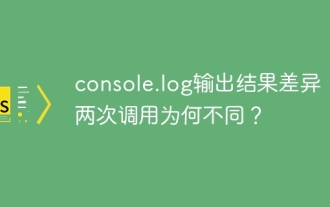
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
