


Here are a few question-based titles that fit your article: * File Reading Error: Why Can\'t I Assign []byte to a String? * Go Error: \'Cannot Assign []byte to z (Type String)\' - How to H
Error Handling in File Reading: Addressing "Cannot Assign []byte to z (Type String)"
When attempting to read files within a folder, an issue arises related to multiple assignment. Let's investigate this error and provide a solution:
The code attempts to list the files within the "documents" folder and then read each file's contents:
files, _ := ioutil.ReadDir("documents/") for _, f := range files { z := "documents/" + f.Name() fmt.Println(z) z, err := ioutil.ReadFile(z) }
The error occurs because the ioutil.ReadFile function returns two values: the contents of the file as a []byte slice and a potential error. However, the code is attempting to assign both values to the same variable z, which is declared as a string.
To resolve this issue, handle the return values correctly:
buf, err := ioutil.ReadFile(z) if err != nil { log.Fatal(err) } z = string(buf)
This separates the conversion to a string from the potential error handling, ensuring that the type mismatch issue is avoided.
Alternatively, to avoid converting to a string, consider working directly with the buf as a binary data representation, reducing unnecessary conversions and potentially improving efficiency in some cases.
The above is the detailed content of Here are a few question-based titles that fit your article: * File Reading Error: Why Can\'t I Assign []byte to a String? * Go Error: \'Cannot Assign []byte to z (Type String)\' - How to H. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










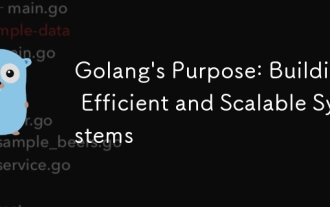
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
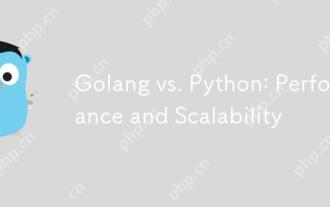
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
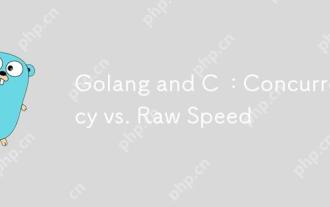
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
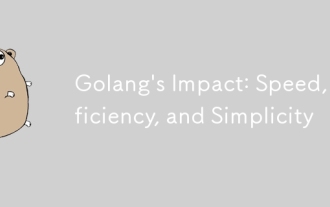
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
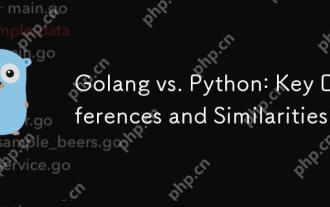
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
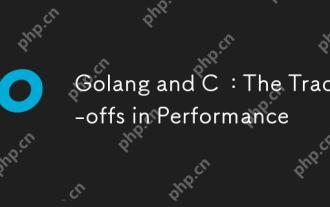
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
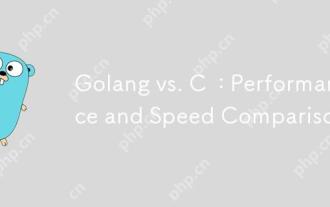
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
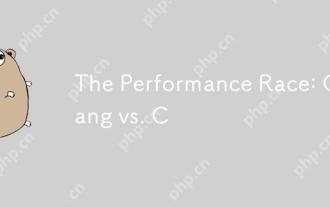
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
