How to Make jQuery Drag/Resize Work with CSS Transform Scale?
Oct 26, 2024 pm 08:18 PMjQuery Drag/Resize with CSS Transform Scale
This question centers around the issue of applying jQuery draggable and resizable plugins to child elements within a div that has a CSS transform (matrix) applied to it. The problem arises when the child elements are dragged or resized, as the adjustments made by jQuery are out of sync with the mouse's position by a factor corresponding to the applied scale.
The Solution
After some experimentation, the user found a solution that involved patching jQuery's draggable and resizable plugins. Here's a summary of the patched code:
- Monkey Patching mouseStart(): This patch ensures that the position of the helper element is calculated correctly by adjusting the mouse offset based on the element's transform scale.
- getViewOffset(): This function assists in correctly calculating the offset of the element under the applied transform.
The jQuery Drag/Resize Patch
The following code demonstrates the modification to the mouseStart() and generatePosition() functions in jQuery UI's draggable plugin:
function monkeyPatch_mouseStart() { // Monkey patch mouseStart function $.ui.draggable.prototype._mouseStart = function(event) { // Calculate adjusted offset this.offset = this.positionAbs = getViewOffset(this.element[0]); } } function generatePosition(event) { // Calculate adjusted position this.top = ( pageY // Absolute mouse position - this.offset.click.top // Click offset - this.offset.relative.top // Element's initial position + ($.browser.safari &amp;&amp; $.browser.version < 526 &amp;&amp; this.cssPosition == 'fixed' ? 0 : ( this.cssPosition == 'fixed' ? -this.scrollParent.scrollTop() : ( this.scrollParent.scrollTop() ) )) ); this.left = ( pageX // Absolute mouse position - this.offset.click.left // Click offset - this.offset.relative.left // Element's initial position + ($.browser.safari &amp;&amp; $.browser.version < 526 &amp;&amp; this.cssPosition == 'fixed' ? 0 : ( this.cssPosition == 'fixed' ? -this.scrollParent.scrollLeft() : scrollIsRootNode ? 0 : this.scrollParent.scrollLeft() )) ); }
Alternative to jQuery
For those seeking alternatives to jQuery for handling drag and resize functionality, here are a few options to consider:
- Vanilla JavaScript: You can implement drag-and-drop functionality using native JavaScript events.
- React.js: React provides a set of hooks like useDrag and useResize for managing these interactions.
- Vue.js: Vuex supports drag-and-drop interactions through the useDrag() hook.
Further Improvement
The user also provides a solution involving callback handlers within the jQuery plugins. This method does not require patching the plugin code and utilizes scale factors to adjust the width, height, and position during drag and resize operations.
// Resizable callback handler $(this).resizable({ ... resize: function(event, ui) { // Adjust width and height relative to scale ui.size.width = ui.originalSize.width + (ui.size.width - ui.originalSize.width) / zoomScale; ui.size.height = ui.originalSize.height + (ui.size.height - ui.originalSize.height) / zoomScale; } }); // Draggable callback handler $(this).draggable({ ... drag: function(event, ui) { // Adjust position relative to scale ui.position.left = ui.originalPosition.left + (ui.position.left - ui.originalPosition.left) / zoomScale; ui.position.top = ui.originalPosition.top + (ui.position.top - ui.originalPosition.top) / zoomScale; } });
This alternative method is a convenient solution for managing drag and resize events in elements with applied transform scales without modifying the jQuery plugins themselves.
The above is the detailed content of How to Make jQuery Drag/Resize Work with CSS Transform Scale?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
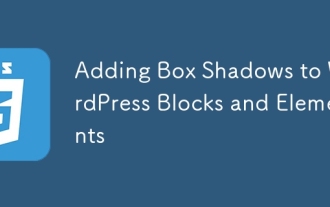
Adding Box Shadows to WordPress Blocks and Elements
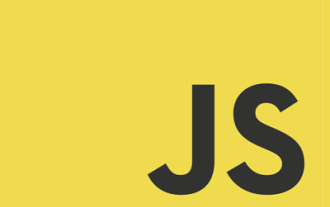
Create a JavaScript Contact Form With the Smart Forms Framework
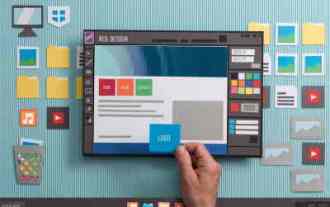
Create an Inline Text Editor With the contentEditable Attribute
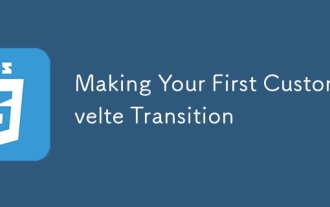
Making Your First Custom Svelte Transition
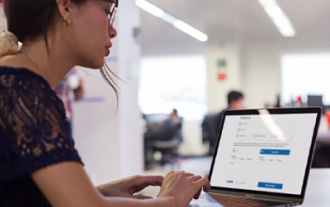
Comparing the 5 Best PHP Form Builders (And 3 Free Scripts)
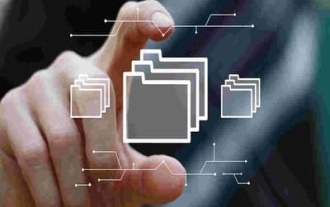
File Upload With Multer in Node.js and Express
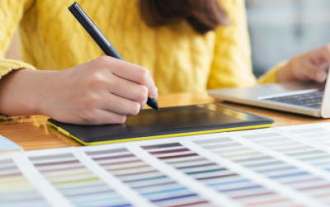
Best CSS Animations and Effects on CodeCanyon 2025 (Paid Free)
