How to Integrate Go Functions into Existing C Projects Using GCCGO?
Using Go Within Existing C Projects
Background:
You have a C program comprised of multiple object files stored in an archive file (.a). You intend to add a new Go file to the project, compile it into an object file, and incorporate it into the existing archive.
Objective:
Integrate Go functions into your C program.
Process:
1. Compile the Go File into an Object File:
-
Run the following command:
gccgo -c printString.go -o printString.o -fgo-prefix=print -Wall -Werror -march=native
Copy after login
2. Call Go Functions from C:
-
In your c_caller.c file, declare an extern function:
extern int PrintString(char*) __asm__ ("print.main.PrintString");
Copy after login -
In the main function, call the Go function and handle the result:
int result = PrintString(string_to_pass); if (result) { printf("Everything went as expected!\n"); } else { printf("Uh oh, something went wrong!\n"); }
Copy after login
3. Using GCCGO to Build the Entire Project:
-
Run the following command:
gccgo -o main c_caller.c printString.o -Wall -Werror -march=native
Copy after login
4. Addressing Errors:
- If you encounter errors like "undefined reference to main.main", ensure that you have a main function in your C code.
- If you encounter errors like "cannot find -lgo," verify that the LD_LIBRARY_PATH environment variable is set to point to the folder where libgo.so is located.
Alternative Solution in Go 1.5:
In Go 1.5 (coming in August), a new feature enables the creation of C-compatible libraries from Go code. With this feature, you can build a static or shared library directly from a Go file, eliminating the need for intermediate object files.
Example:
-
In main.c:
#include <stdio.h> int main() { char *string_to_pass = NULL; if (asprintf(&string_to_pass, "This is a test.") < 0) { printf("asprintf fail"); return -1; } PrintString(string_to_pass); return 0; }
Copy after login -
In main.go (compiled with go build -buildmode c-archive ... for static or go build -buildmode c-shared ... for shared library):
package main import "C" import "fmt" //export PrintString func PrintString(cs *C.char) { s := C.GoString(cs) fmt.Println(s) } func main() {}
Copy after login
The above is the detailed content of How to Integrate Go Functions into Existing C Projects Using GCCGO?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










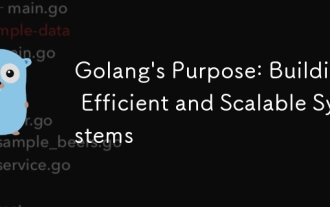
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
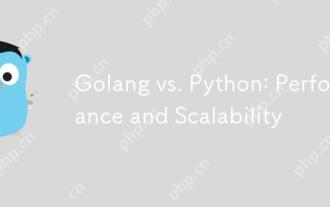
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
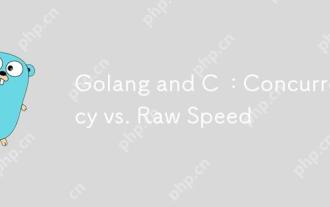
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
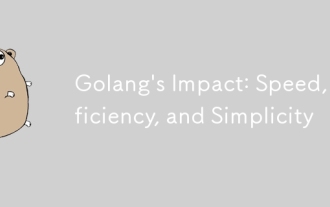
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
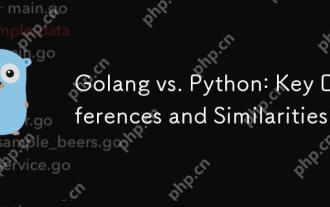
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
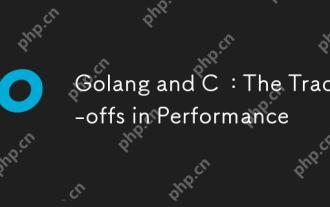
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
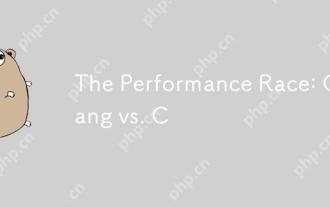
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
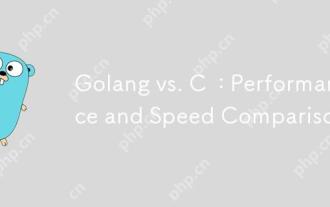
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
