


How to Implement a Grid Structure for Pixel Editor Using `drawImage()` Method?
Grid Implementation for Pixel Editor
In the development of a pixel editor, determining an effective method for implementing a grid structure that accommodates color changes when clicked and dragged is essential. A typical approach involves employing individual JButtons for each cell, but this may be inefficient and cumbersome.
Grid with Image Scaling
An alternative solution involves using the drawImage() method to create large pixels by scaling the mouse coordinates. This technique provides a simple and efficient means of altering the color of multiple cells simultaneously, as demonstrated below:
<code class="java">import java.awt.Dimension; import java.awt.EventQueue; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Point; import java.awt.event.MouseEvent; import java.awt.event.MouseMotionListener; import java.awt.image.BufferedImage; import javax.swing.Icon; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.UIManager; /** @see http://stackoverflow.com/questions/2900801 */ public class Grid extends JPanel implements MouseMotionListener { private final BufferedImage img; private int imgW, imgH, paneW, paneH; public Grid(String name) { super(true); Icon icon = UIManager.getIcon(name); imgW = icon.getIconWidth(); imgH = icon.getIconHeight(); this.setPreferredSize(new Dimension(imgW * 10, imgH * 10)); img = new BufferedImage(imgW, imgH, BufferedImage.TYPE_INT_ARGB); Graphics2D g2d = (Graphics2D) img.getGraphics(); icon.paintIcon(null, g2d, 0, 0); g2d.dispose(); this.addMouseMotionListener(this); } @Override protected void paintComponent(Graphics g) { paneW = this.getWidth(); paneH = this.getHeight(); g.drawImage(img, 0, 0, paneW, paneH, null); } @Override public void mouseMoved(MouseEvent e) { Point p = e.getPoint(); int x = p.x * imgW / paneW; int y = p.y * imgH / paneH; int c = img.getRGB(x, y); this.setToolTipText(x + "," + y + ": " + String.format("%08X", c)); } @Override public void mouseDragged(MouseEvent e) { } private static void create() { JFrame f = new JFrame(); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.add(new Grid("Tree.closedIcon")); f.pack(); f.setVisible(true); } public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { create(); } }); } }</code>
By utilizing this technique, you can efficiently implement a customizable grid system with adjustable color properties, meeting the requirements of your pixel editor application.
The above is the detailed content of How to Implement a Grid Structure for Pixel Editor Using `drawImage()` Method?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










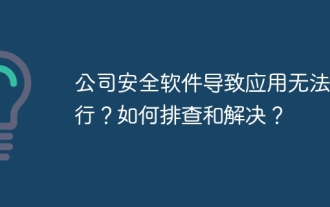
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
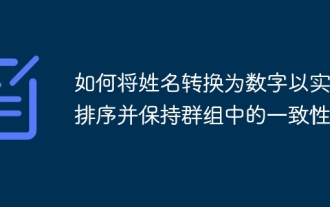
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
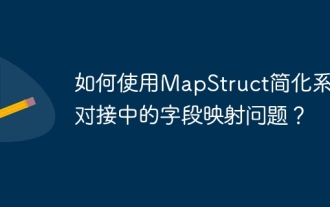
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
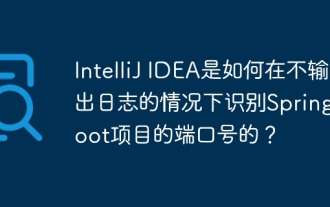
Start Spring using IntelliJIDEAUltimate version...
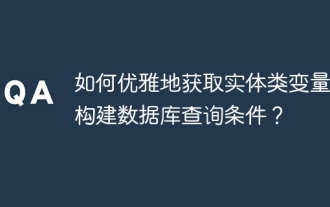
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
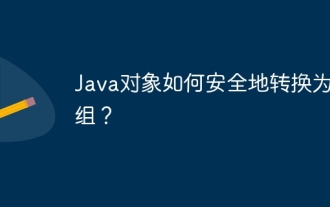
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
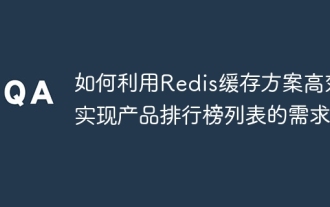
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
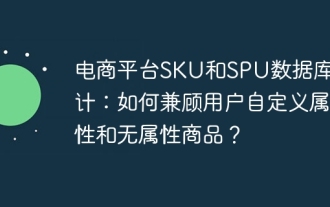
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
