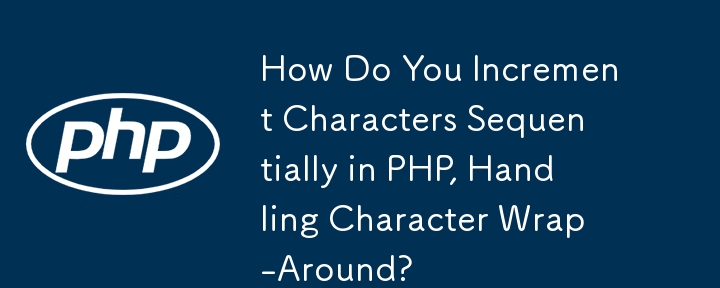
Incrementing Characters Sequentially in PHP
In PHP, incrementing or decrementing characters is an uncommon but useful operation. This article addresses the challenge of incrementing a string of characters like numeric values, considering the complexities when characters wrap from 'Z' to 'A.'
Understanding the Logic
To increment a string of characters sequentially, we need a way to monitor when the last character has reached the end of the alphabet and determine when to move on to the next character. Here's the logic used:
- If the current character is not 'Z,' increment it by 1.
- If the current character is 'Z' and the previous character is not 'Z,' increment the previous character by 1 and reset the current character to 'A.'
- If both the current and previous characters are 'Z,' increment the character before the previous one by 1 and reset both the previous and current characters to 'A.'
- Continue this process recursively until no more characters are left to increment.
PHP Functions for Character Manipulation
PHP provides several useful functions for manipulating characters:
-
ord(): Returns the ASCII code of a character.
-
chr(): Converts an ASCII code to a character.
-
strlen(): Returns the length of a string.
-
substr(): Extracts a part of a string.
The PHP Function for Character Increment
Here's a PHP function that implements the described logic:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | <code class = "php" > function increment_chars( $str ) {
$len = strlen ( $str );
$arr = array_map ( 'ord' , str_split ( $str ));
$index = $len - 1;
while ( $index >= 0) {
if ( $arr [ $index ] < 90) {
$arr [ $index ]++;
break ;
}
else {
$arr [ $index ] = 65;
$index --;
}
}
$result = "" ;
foreach ( $arr as $ascii ) {
$result .= chr ( $ascii );
}
return $result ;
}</code>
|
Copy after login
Usage Example
To increment the string "AAZ," we can use the function as follows:
1 2 3 | <code class = "php" > $str = "AAZ" ;
$incremented_str = increment_chars( $str );
echo $incremented_str ;
|
Copy after login
The above is the detailed content of How Do You Increment Characters Sequentially in PHP, Handling Character Wrap-Around?. For more information, please follow other related articles on the PHP Chinese website!