Why does shuffling a slice affect a slice assigned to it in Go?
Array Handling in Go
In Go, arrays are value types, and assigning one array to another creates a copy of all its elements. This holds true even when passing an array to a function, as it will receive a copy rather than a memory reference.
Original Question
A query was raised regarding the following code:
<code class="go">package main import ( "fmt" "rand" "time" ) func shuffle(arr []int) { rand.Seed(time.Nanoseconds()) for i := len(arr) - 1; i > 0; i-- { j := rand.Intn(i) arr[i], arr[j] = arr[j], arr[i] } } func main() { arr := []int{1, 2, 3, 4, 5} arr2 := arr shuffle(arr) for _, i := range arr2 { fmt.Printf("%d ", i) } }</code>
The author expressed confusion as to why arr2 was affected by the shuffle function, despite their expectation of arr2 and arr being distinct entities.
Clarification
The issue stems from a misunderstanding between arrays and slices.
Arrays vs Slices
Arrays are fixed-length collections of values, while slices are dynamic references to underlying arrays. In the provided code example, no arrays are used.
Slice Manipulation
The arr := []int{1, 2, 3, 4, 5} line creates a slice referencing an anonymous underlying array. The subsequent arr2 := arr line simply duplicates this reference, resulting in both arr and arr2 pointing to the same underlying array.
Function Behavior
When passing arr to the shuffle function, a copy of the slice is created, not the underlying array. This copy is modified by the function, which is why arr2 is also affected when arr is modified.
Conclusion
In Go, slices behave as references to underlying arrays. Assigning one slice to another copies the reference, not the underlying array. This concept is crucial for understanding slice manipulation in Go.
The above is the detailed content of Why does shuffling a slice affect a slice assigned to it in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










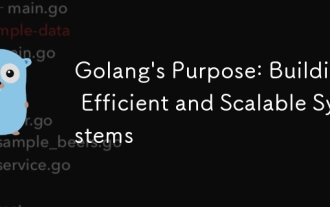
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
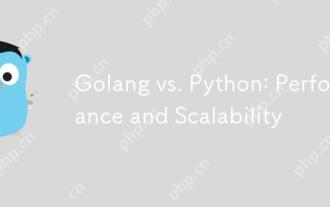
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
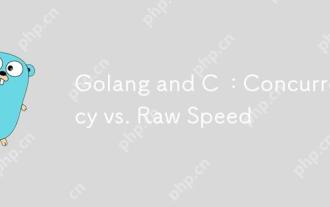
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
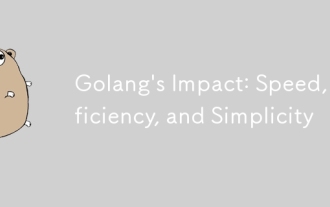
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
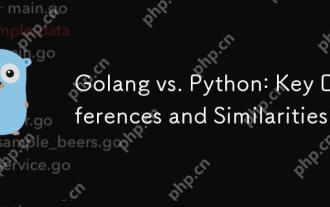
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
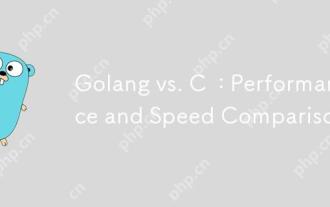
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
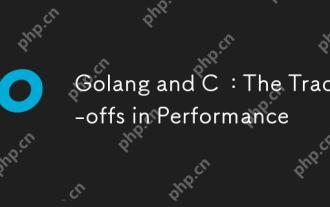
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
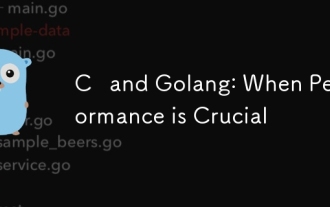
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
