


How to Split a Large Pandas Dataframe into Multiple Parts When the Number of Rows is Not Evenly Divisible?
Splitting Large Pandas Dataframes into Multiple Parts
When working with massive datasets, it often becomes necessary to split them into smaller, manageable chunks. This can improve performance, enhance memory usage, and facilitate parallel processing. In this article, we'll address an encountered issue while attempting to split a large pandas dataframe using np.split().
Understanding the Issue
The provided code snippet employed np.split() to partition a dataframe into four subgroups. However, it resulted in a ValueError due to an unequal division. This error arises when the number of elements in the dataframe is not evenly divisible by the desired number of splits.
Solution: Using np.array_split()
To overcome this challenge, we employ np.array_split(), a more versatile alternative to np.split(). As its documentation states, array_split() allows for non-equal division, making it suitable for situations like ours.
Implementation
Here's a Python code example using np.array_split() to split the dataframe into four parts:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
This will effectively partition the dataframe into three approximately equal-sized groups. Each group can be accessed and processed independently, addressing the initial challenge of unequal division.
The above is the detailed content of How to Split a Large Pandas Dataframe into Multiple Parts When the Number of Rows is Not Evenly Divisible?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
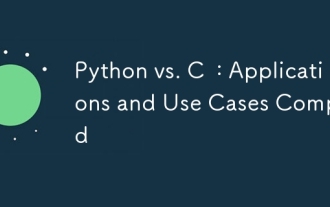
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
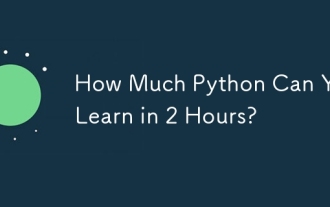
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
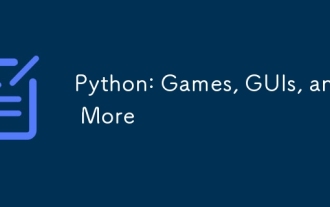
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
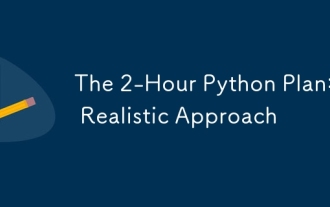
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
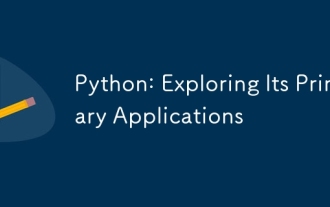
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
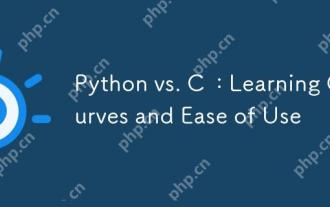
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
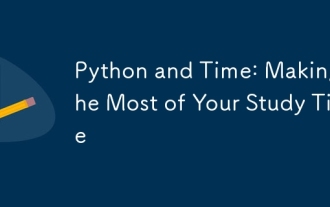
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
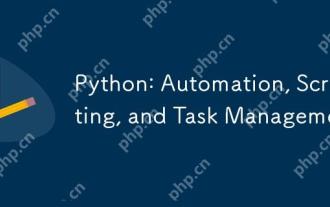
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
