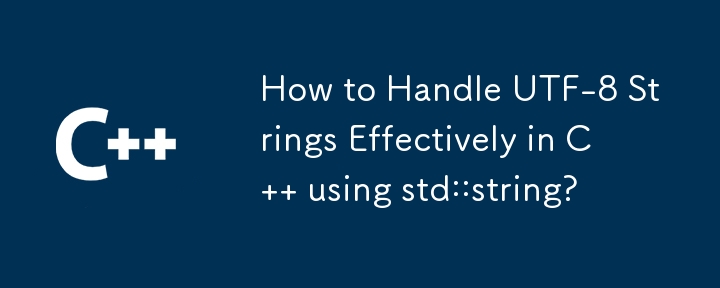
Handling UTF-8 in C Using std::string
Background Information
Unicode: Unicode is an international standard for encoding characters of various languages and scripts.
Code Points and Grapheme Clusters: Unicode characters are mapped to code points, and groups of code points may form grapheme clusters (e.g., certain diacritics).
UTF Encodings: UTF-8, UTF-16, and UTF-32 are common Unicode encodings, where X represents the number of bits per code unit.
std::string and std::wstring for Unicode
std::wstring Limitations: wchar_t is typically 16 bits on Windows, which may not adequately represent all code points. Consider std::u32string (std::basic_string) instead.
Memory Representation and Conversion: The in-memory representation (std::string or std::wstring) differs from the on-disk representation (e.g., UTF-8), so conversion may be required.
Handling UTF-8 in std::string
Advantages:
- Smaller memory footprint due to 8-bit code units.
- Backward compatible with ASCII.
Considerations:
- std::string::size() returns the number of bytes, not code points.
- Operations like str[i] may access individual bytes, not code points.
- Use std::string::substr(n, width) to retrieve a substring of a specific width (in bytes).
- Regex may not correctly handle character classes or repetitions for non-ASCII characters. Use parentheses to specify the repeated sequence explicitly.
Choosing Between std::string and std::u32string
-
Performance: std::string may be more performant.
-
Grapheme Clusters: std::u32string simplifies grapheme cluster handling.
-
Interfacing with Other Software: Use std::string if interfacing with software that uses std::string or char/char const.
Handling Grapheme Clusters in UTF-8
-
Consider Unicode-aware Libraries: Libraries like ICU can handle grapheme clusters effectively.
-
Use Iterators: Iterate over code points rather than bytes using iterators, such as std::string::begin() and std::string::end().
-
Encode and Decode Surrogate Pairs: For extended code points that span multiple bytes, encode them as surrogate pairs and decode them for proper handling.
The above is the detailed content of How to Handle UTF-8 Strings Effectively in C using std::string?. For more information, please follow other related articles on the PHP Chinese website!