


How to Efficiently Index a 2D NumPy Array Using Two Lists of Indices?
Indexing a 2D NumPy Array Using Two Lists of Indices
The objective is to perform indexing on a 2D NumPy array using two provided lists of indices, one for rows and one for columns. The desired outcome is to obtain a subset of the array based on the specified indices efficiently.
Utilizing np.ix_
To achieve this, we can leverage the np.ix_ function from NumPy. np.ix_ creates tuples of indexing arrays that can be employed for broadcasting. Here's how it works:
With Indexing Arrays
Selection:
<code class="python">x_indexed = x[np.ix_(row_indices, col_indices)]</code>
This creates a tuple of indexing arrays based on row_indices and col_indices. Broadcasting these arrays enables us to index into x and extract the desired subset.
Assignment:
<code class="python">x[np.ix_(row_indices, col_indices)] = value</code>
This assigns the specified value into the indexed positions in x.
With Masks
Selection:
<code class="python">row_mask = np.array([True, False, False, True, False], dtype=bool) col_mask = np.array([False, True, True, False, False], dtype=bool) x_indexed = x[np.ix_(row_mask, col_mask)]</code>
Here, we use boolean masks (row_mask and col_mask) to define which rows and columns to select.
Assignment:
<code class="python">x[np.ix_(row_mask, col_mask)] = value</code>
This assigns value to the masked positions in x.
Sample Run
Consider the following array and index lists:
<code class="python">x = np.random.random_integers(0, 5, (20, 8)) row_indices = [4, 2, 18, 16, 7, 19, 4] col_indices = [1, 2]</code>
Using np.ix_, we can index into x:
<code class="python">x_indexed = x[np.ix_(row_indices, col_indices)] print(x_indexed) # Output: # [[76 56] # [70 47] # [46 95] # [76 56] # [92 46]]</code>
This gives us the desired subset of the array with rows and columns selected based on the provided indices.
The above is the detailed content of How to Efficiently Index a 2D NumPy Array Using Two Lists of Indices?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


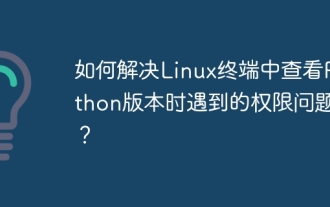
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
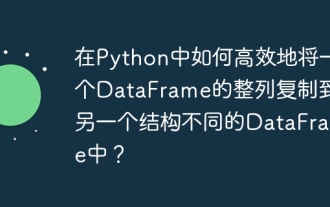
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
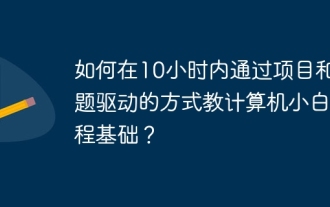
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
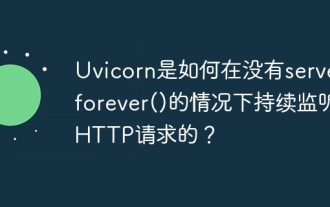
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
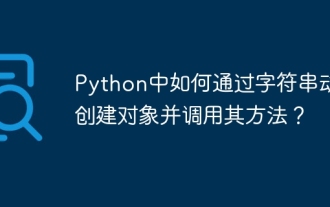
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
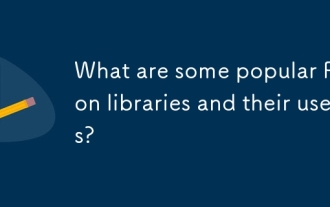
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
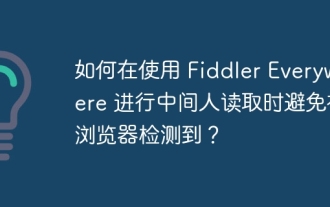
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
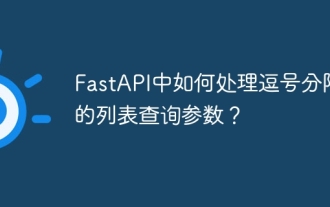
Fastapi ...
