


The TypeScript Intervention: Breaking Your Runtime Check Addiction with Byzantium
Oct 27, 2024 am 06:15 AMLook, we need to talk about your type checking addiction. Yes, you – the one with 47 instanceof checks in your authentication middleware. The developer who writes more test cases than actual code. The one who treats TypeScript like it's just fancy JSDoc comments.
The Intervention
Let me paint you a picture: It's midday, you're on your 4th cup of coffee, and you're debugging a production issue. The logs show a user somehow got past your fifteen layers of runtime validation. You've got more unit tests than Twitter has active users, yet somehow, somehow, someone managed to send a number where a string should be.
"But that's impossible!" you cry, scrolling through your test coverage report showing a pristine 100%. "I checked for this!"
Did you though? Did you really? Or did you just write the same check three times:
- Once in TypeScript interfaces
- Again in your validation middleware
- And yet again in your unit tests
Stop Testing What TypeScript Already Knows
Here's a revolutionary idea: What if we just... trusted the compiler? I know, wild concept. But hear me out.
interface ValidRabbit { username: string; password: string; } interface InvalidRabbit { username: number; password: string; } type ValidateRabbit<Rabbit> = Assert< //assert that Rabbit is of type {username, password} Is.Type< User, { username: string; password: string; } >, //custom compile time exceptions "Trix are for kids. Provide a username and password.", User >; // Ha! Silly Rabbit... function checkRabbit<T>(rabbit: ValidateRabbit<T>) { // .... protect your trix } declare const rabbit1: ValidRabbit; declare const rabbit2: InvalidRabbit; checkRabbit(rabbit1); checkRabbit(rabbit2); /** ~~~~~~~~~ * └───── Type Exception! "...Provide a username and password" */
"But What About Production?"
I can hear you now: "But what if someone sends invalid JSON to my API?"
First of all, who hurt you? Second, yes, validate your API boundaries. But once that data enters your typescript domain, it's time to let go. Let the compiler be your bouncer.
Here's what Byzantium brings to your trust issues party:
// Define your trust boundaries type APIRequest<Request> = Assert< And< Is.On<Request, "body">, Or<Is.In<Request["method"], "POST">, Is.In<Request["method"], "PUT">> >;, "Someone's being naughty with our API" >; // Now everything inside is type-safe function handleRequest<R>(req: APIRequest<R>) { // If it compiles, it's valid // If it's valid, it compiles // This is the way }
The DevOps Team Will Love You (For Once)
Picture this: Your CI/CD pipeline finishes in minutes instead of hours. Your production logs aren't filled with type errors. Your AWS bill doesn't look like a phone number.
How? Because Byzantium moves your type checking to compile time. No more:
- Running thousands of unit tests that just check types
- Consuming CPU cycles checking the same types over and over
- Waking up at 3 AM because someone passed undefined to a function that clearly said it wanted a string
// Before: Your CPU crying for help function validateUserMiddleware(req, res, next) { try { validateId(req.params.id) // CPU cycle validateBody(req.body) // CPU cycle validatePermissions(req.user) // CPU cycle validateToken(req.headers.auth) // CPU cycle // Your CPU is now considering a career change next() } catch (e) { res.status(400).json({ error: e.message }) } } // After: Your CPU sending you a thank you note type ValidRequest = Assert< And< Is.On<Request, 'params.id'>, Is.On<Request, 'body'>, Is.On<Request, 'user'>, Is.On<Request, 'headers.auth'> >, "Invalid request shape" >; function handleRequest(req: ValidRequest) { // Just business logic, no trust issues }
"But I Love Writing Tests!"
Great! Write tests for things that actually need testing:
- Business logic
- Integration points
- User workflows
- Complex algorithms
You know what doesn't need testing? Whether a string is actually a string. Let TypeScript handle that existential crisis.
Real Talk: The Benefits
-
Faster Development
- No more writing the same validation three different ways
- Catch errors at compile time, not at 3 AM
- Spend time on features, not validation boilerplate
-
Better Performance
- Zero runtime overhead for type checking
- Smaller bundle sizes (no validation libraries)
- Happy CPUs, happy life
-
Improved Security
- Type-level guarantees can't be bypassed
- No more "oops, forgot to validate that"
- Complete coverage by default
-
DevOps Dreams
- Faster CI/CD pipelines
- Lower infrastructure costs
- Fewer production incidents
- Happier SRE team (results may vary)
Getting Started
interface ValidRabbit { username: string; password: string; } interface InvalidRabbit { username: number; password: string; } type ValidateRabbit<Rabbit> = Assert< //assert that Rabbit is of type {username, password} Is.Type< User, { username: string; password: string; } >, //custom compile time exceptions "Trix are for kids. Provide a username and password.", User >; // Ha! Silly Rabbit... function checkRabbit<T>(rabbit: ValidateRabbit<T>) { // .... protect your trix } declare const rabbit1: ValidRabbit; declare const rabbit2: InvalidRabbit; checkRabbit(rabbit1); checkRabbit(rabbit2); /** ~~~~~~~~~ * └───── Type Exception! "...Provide a username and password" */
The Choice is Yours
You can keep living in fear, writing runtime checks for everything, treating TypeScript like it's optional typing for JavaScript.
Or you can join us in 2024, where we trust our compiler and let it do its job.
Remember: Every time you write a runtime type check, somewhere a TypeScript compiler cries.
Conclusion
Byzantium isn't just another library – it's an intervention for your trust issues with types. It's time to let go of the runtime checks and embrace the power of compile-time guarantees.
Your CPU will thank you. Your DevOps team will thank you. Your users will thank you (by not finding type-related bugs).
And most importantly, you'll thank yourself at 3 AM when you're sleeping soundly instead of debugging type errors in production.
P.S. If you're still not convinced, try counting how many runtime type checks you have in your codebase. Then multiply that by your hourly rate. That's how much time you're spending not trusting TypeScript.
P.P.S. No types were harmed in the making of this blog post. Though several runtime checks were permanently retired.
*P.P.P.S. If you would like to contribute, stop by my Github and clone the repo. Everything is still fresh, so lots of opportunity to contribute.
Docs and Package available on JSR.io
The above is the detailed content of The TypeScript Intervention: Breaking Your Runtime Check Addiction with Byzantium. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
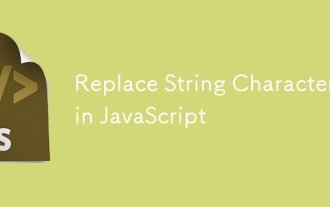
Replace String Characters in JavaScript
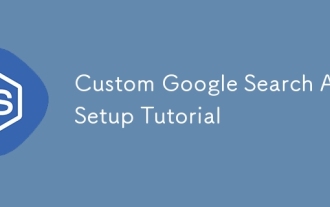
Custom Google Search API Setup Tutorial
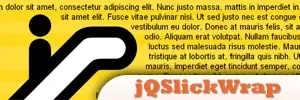
8 Stunning jQuery Page Layout Plugins
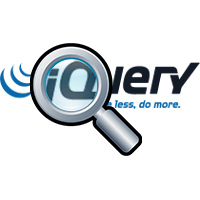
Improve Your jQuery Knowledge with the Source Viewer
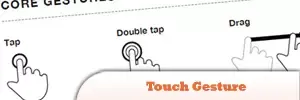
10 Mobile Cheat Sheets for Mobile Development
