


How to Create a FastAPI Endpoint That Accepts Either Form or JSON Body?
How to create a FastAPI endpoint that can accept either Form or JSON body?
In FastAPI, you can define endpoints that handle various types of request bodies, such as JSON or form-data. This allows you to create endpoints that can accept either format without the need for separate endpoints.
To achieve this, you can follow one of the below approaches:
Option 1: Using a dependency function
You can utilize a dependency function to verify the request's Content-Type header, then parse the body appropriately using Starlette's methods. Note that relying solely on the Content-Type header may not always guarantee the validity of the request body, so it's recommended to include error handling.
<code class="python">import os, sys from fastapi import FastAPI, Depends, HTTPException from starlette.requests import Request app = FastAPI() # Generating file open("./app.txt", "w").write("hello from a file") async def body_parser(request: Request): ct = request.headers.get("Content-Type", "") if ct == "application/json": try: d = await request.json() if not isinstance(d, dict): raise HTTPException(status_code=400, details={"error":"request body must be a dict"}) return d except JSONDecodeError: raise HTTPException(400, "Could not parse request body as JSON") elif ct == "multipart/form-data": await request.stream() # this is required for body parsing. d = await request.form() if not d: raise HTTPException(status_code=400, details={"error":"no form parameters found"}) return d else: raise HTTPException(405, "Content-Type must be either JSON or multipart/form-data") @app.post("/", dependencies=[Depends(body_parser)]) async def body_handler(d: dict): if "file" in d: return {"file": d["file"]} return d</code>
Option 2: Utilizing optional form/file parameters
In this approach, you can define form/file parameters as optional in your endpoint. If any of these parameters have values, it assumes a form-data request. Otherwise, it validates the request body as JSON.
<code class="python">from fastapi import FastAPI, Form, File, UploadFile app = FastAPI() @app.post("/") async def file_or_json( files: List[UploadFile] = File(None), some_data: str = Form(None) ): if files: return {"files": len(files)} return {"data": some_data}</code>
Option 3: Defining separate endpoints for each type
You can also create separate endpoints, one for JSON and another for form-data. Using a middleware, you can check the Content-Type header and reroute the request to the appropriate endpoint.
<code class="python">from fastapi import FastAPI, Request, Form, File, UploadFile from fastapi.responses import JSONResponse app = FastAPI() @app.middleware("http") async def middleware(request: Request, call_next): ct = request.headers.get("Content-Type", "") if ct == "application/json": request.scope["path"] = "/json" elif ct in ["multipart/form-data", "application/x-www-form-urlencoded"]: request.scope["path"] = "/form" return await call_next(request) @app.post("/json") async def json_endpoint(json_data: dict): pass @app.post("/form") async def form_endpoint(file: UploadFile = File(...)): pass</code>
Option 4: Referencing another answer for an alternative approach
Additionally, you may find this answer on Stack Overflow helpful as it provides a different perspective on handling both JSON and form-data in a single endpoint:
https://stackoverflow.com/a/67003163/10811840
Testing Options 1, 2, & 3
For testing purposes, you can use requests library:
<code class="python">import requests url = "http://127.0.0.1:8000" # for testing Python 3.7 and above use: # url = "http://localhost:8000" # form-data request files = [('files', ('a.txt', open('a.txt', 'rb'), 'text/plain'))] response = requests.post(url, files=files) print(response.text) # JSON request data = {"some_data": "Hello, world!"} response = requests.post(url, json=data) print(response.text)</code>
These approaches provide different methods to create an endpoint that can handle both JSON and form-data in FastAPI. Choose the approach that best fits your requirements and use case.
The above is the detailed content of How to Create a FastAPI Endpoint That Accepts Either Form or JSON Body?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










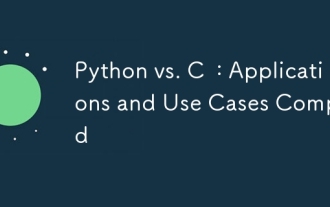
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
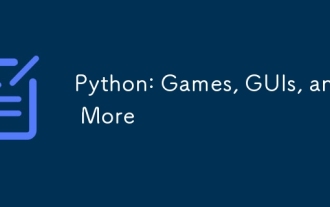
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
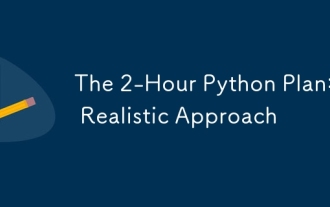
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
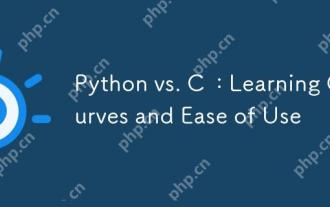
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
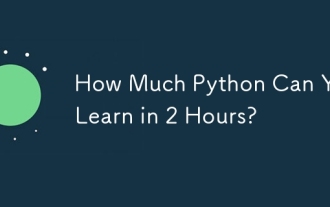
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
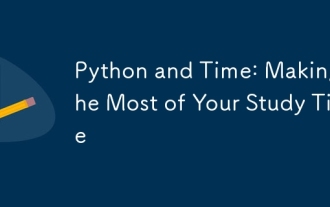
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
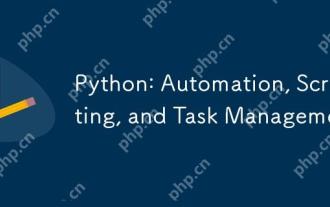
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
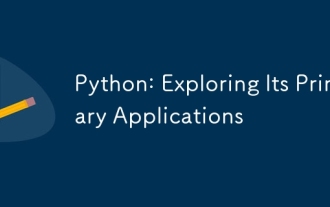
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
