


How Can You Improve Scripting Engine Efficiency with STL Maps for Function Pointer Management?
Incorporating STL Map into a Scripting Engine for Function Pointer Storage
To enhance the efficiency of your scripting engine, consider leveraging an STL map for managing function pointers. This approach eliminates the need for lengthy conditional statements to invoke specific functions.
For this implementation, begin by declaring your function pointer type as a typedef for readability:
<code class="c++">typedef void (*ScriptFunction)(void); // function pointer type</code>
Next, define an unordered_map named script_map with string keys representing function names and ScriptFunction values for the corresponding pointer addresses:
<code class="c++">typedef std::unordered_map<std::string, ScriptFunction> script_map;</code>
Example function registration:
<code class="c++">void some_function() {} script_map m; m.emplace("blah", &some_function);</code>
To call a function, define a call_script function:
<code class="c++">void call_script(const std::string& pFunction) { auto iter = m.find(pFunction); if (iter == m.end()) { // function not found } else { (*iter->second)(); // invoke the function via the pointer } }</code>
Emphasize that you can generalize the ScriptFunction type to std::function* whatever*/> to cater to more than just bare function pointers.
The above is the detailed content of How Can You Improve Scripting Engine Efficiency with STL Maps for Function Pointer Management?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


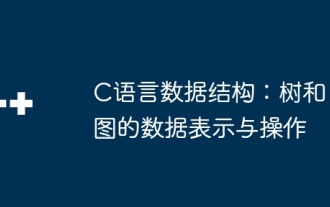
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
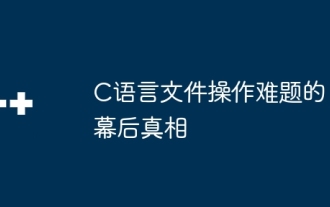
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
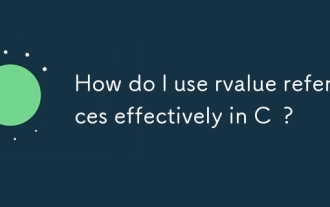
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
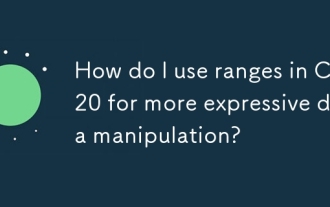
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
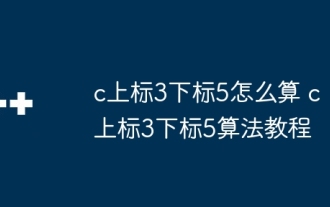
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
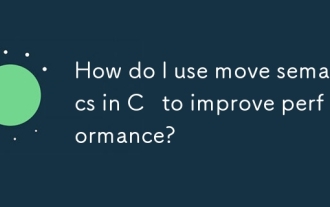
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
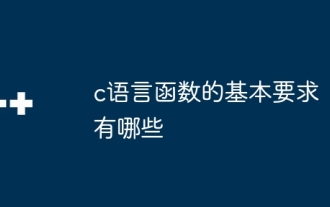
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
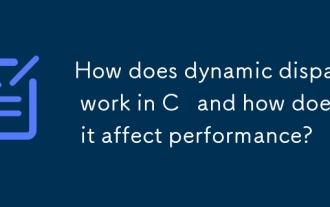
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
