


How to Avoid Code Repetition for Functions with Shared Fields in Golang?
Oct 27, 2024 am 07:50 AMAvoiding Code Repetition for Functions with Shared Fields in Golang
To prevent code duplication when writing functions for multiple structs with identical fields, consider the following approach:
Instead of defining separate functions for each struct, create a custom type for the shared field, such as Version string. This type can act as a method receiver for the function you want to implement.
<code class="go">type Version string func (v Version) PrintVersion() { fmt.Println("Version is", v) }</code>
In your structs, include the custom type as a field using composition:
<code class="go">type Game struct { Name string MultiplayerSupport bool Genre string Version } type ERP struct { Name string MRPSupport bool SupportedDatabases []string Version }</code>
Now, you can access and print the Version field from both structs using the method attached to the custom type:
<code class="go">func main() { g := Game{ "Fear Effect", false, "Action-Adventure", "1.0.0", } g.PrintVersion() // Version is 1.0.0 e := ERP{ "Logo", true, []string{"ms-sql"}, "2.0.0", } e.PrintVersion() // Version is 2.0.0 }</code>
This approach allows you to avoid code repetition while maintaining the ability to print the Version field from different structs. By defining the function as a method of a custom type, you ensure that the same implementation is used for all structs that embed that type.
The above is the detailed content of How to Avoid Code Repetition for Functions with Shared Fields in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
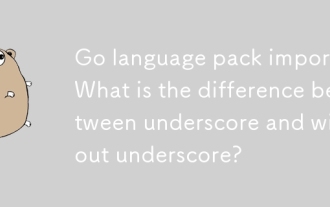
Go language pack import: What is the difference between underscore and without underscore?
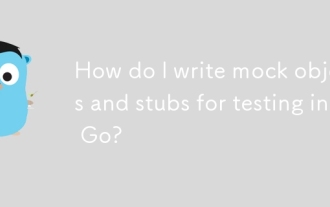
How do I write mock objects and stubs for testing in Go?

How to implement short-term information transfer between pages in the Beego framework?
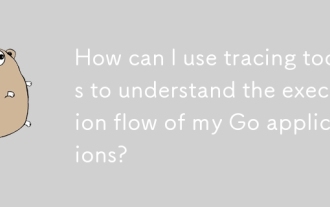
How can I use tracing tools to understand the execution flow of my Go applications?
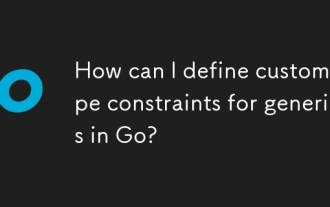
How can I define custom type constraints for generics in Go?
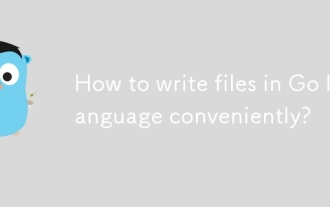
How to write files in Go language conveniently?
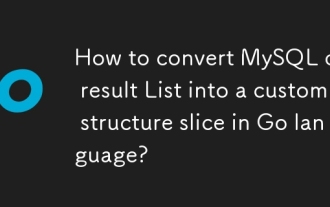
How to convert MySQL query result List into a custom structure slice in Go language?
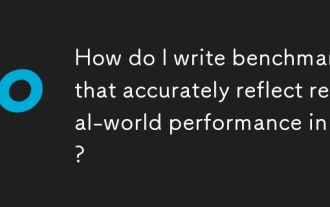
How do I write benchmarks that accurately reflect real-world performance in Go?
