How to Gracefully Interrupt a Blocking ServerSocket.accept() Method?
Oct 27, 2024 pm 06:58 PMInterrupting a Blocking ServerSocket Accept() Method
In a multi-threaded application, it may be necessary to interrupt a blocking method such as ServerSocket.accept(). This is typically required for graceful shutdown or to respond to administrative commands.
The traditional accept() method blocks until a client connection is received. This behavior can hinder the ability to respond to external events or terminate the application promptly.
Solution: Using Close() to Interrupt
One effective solution to interrupt the blocking accept() method is to call close() on the ServerSocket object from a separate thread. When close() is invoked, the accept() method will throw a SocketException.
<code class="java">// In the admin thread ServerSocket serverSocket = ...; serverSocket.close();</code>
Handling the Interruption
Within the main loop where accept() is being called, it is necessary to catch the SocketException and handle it gracefully.
<code class="java">while (listening) { try { // Accept client connection Socket clientSocket = serverSocket.accept(); // ... Process client connection } catch (SocketException e) { // Handle the interruption // ... Shut down client threads, admin thread, and main thread break; } }</code>
Benefits of This Approach
- Allows for graceful shutdown of the application, even while accept() is blocking.
- Provides a reliable way to interrupt the blocking method without having to modify the ServerSocket class itself.
The above is the detailed content of How to Gracefully Interrupt a Blocking ServerSocket.accept() Method?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
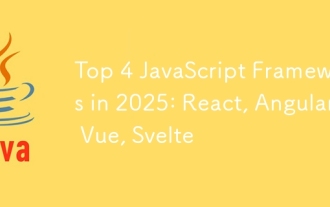
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
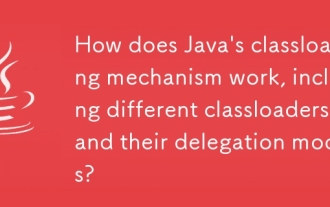
How does Java's classloading mechanism work, including different classloaders and their delegation models?
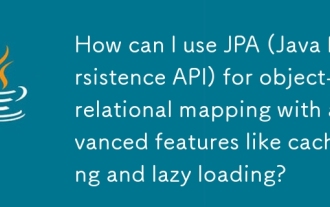
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
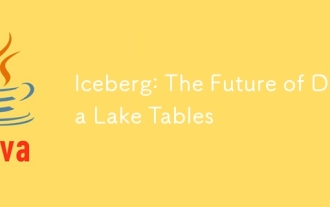
Iceberg: The Future of Data Lake Tables
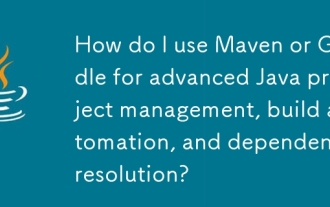
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
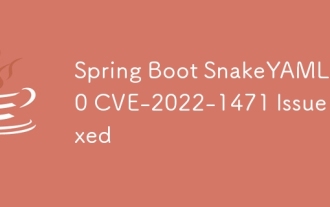
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
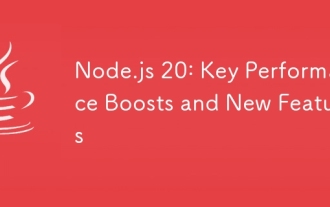
Node.js 20: Key Performance Boosts and New Features
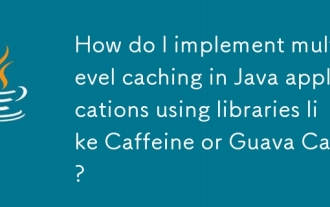
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
