


How to Cast Between a void* Pointer and a Pointer to a Member Function in C ?
Casting between void* and a Pointer to Member Function
When working with C/C interoperability, one can encounter difficulties when attempting to cast between a void pointer and a pointer to a member function. This issue often arises when dealing with closures in languages like Lua, where user data is stored as void pointers.
Problem:
Casting void* to a pointer to a member function in C is invalid due to the fundamental differences in their memory representations. Pointers-to-members contain additional information not accessible through regular pointers.
Solution:
To workaround this, consider the following options:
1. Wrap the Member Function in a Free Function:
- Create a free function that accepts the object instance as its first argument and the member function parameters as subsequent arguments.
- Return the address of this free function instead of the pointer-to-member.
Code Snippet:
<code class="cpp">int call_int_function(lua_State *L) { void (*method)(T*, int, int) = reinterpret_cast<void (*)(T*, int, int)>(lua_touserdata(L, lua_upvalueindex(1))); T *obj = reinterpret_cast<T *>(lua_touserdata(L, 1)); method(obj, lua_tointeger(L, 2), lua_tointeger(L, 3)); return 0; }</code>
2. Use a Function Object:
- Utilize a technique similar to Boost.Bind's mem_fun to create a function object that wraps the member function.
- Template the function object to support different object types.
Other Considerations:
- Ensure control over the user data returned by lua_touserdata as it cannot contain a valid pointer-to-member.
- Consider wrapping the member function in a class method or static function if you require additional state.
The above is the detailed content of How to Cast Between a void* Pointer and a Pointer to a Member Function in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
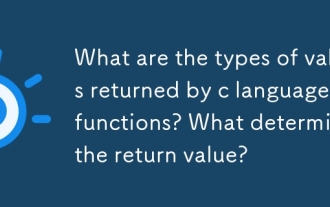
What are the types of values returned by c language functions? What determines the return value?
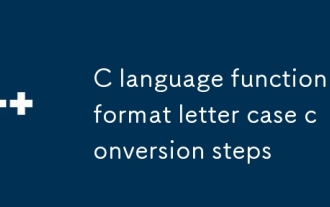
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
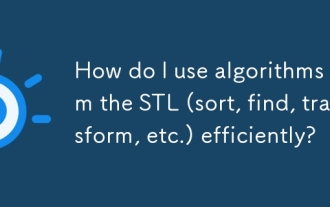
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
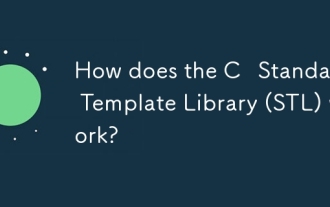
How does the C Standard Template Library (STL) work?
