


How can I reliably download a PDF file from a web service response across different browsers using JavaScript?
Constructing PDF Documents from Web Service Responses in JavaScript
Building PDF documents from binary strings returned by web services presents a cross-browser challenge. The data-uri method, while effective in certain browsers like Chrome and Opera, falls short in IE9 and Firefox.
Alternative Approach: Client-Side File Download
To overcome these limitations, an alternative approach involves creating PDF files on the file system for user download. To accomplish this, consider the following:
Setting Response Type to Blob
Configure the responseType property of the XMLHttpRequest object to "blob" instead of "text". This allows the client to receive the PDF data as a Blob object.
Utilizing the Blob Object
Create a new Blob object using the response obtained from the web service request.
Generating an Object URL
Generate an object URL for the Blob object, which represents the PDF file.
Creating a Download Link
Triggering the Download
Append the element to the document body and click on it to initiate the file download.
Example Code
The following JavaScript code demonstrates this approach:
<code class="javascript">var request = new XMLHttpRequest(); request.open("GET", "/path/to/pdf", true); request.responseType = "blob"; request.onload = function (e) { if (this.status === 200) { // Generate .pdf name as `Blob` from `this.response` var file = window.URL.createObjectURL(this.response); var a = document.createElement("a"); a.href = file; a.download = this.response.name || "detailPDF"; document.body.appendChild(a); a.click(); // Remove `a` after `Save As` dialog window.onfocus = function () { document.body.removeChild(a); }; }; }; request.send();</code>
This method ensures a reliable file download across multiple browsers, including IE9, Firefox, Chrome, Opera, and Safari.
The above is the detailed content of How can I reliably download a PDF file from a web service response across different browsers using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
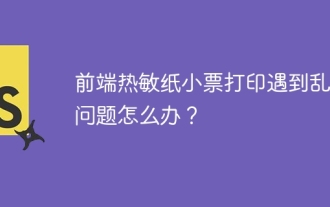
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
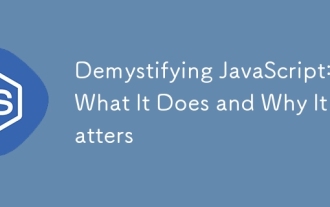
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
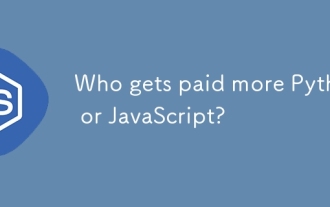
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
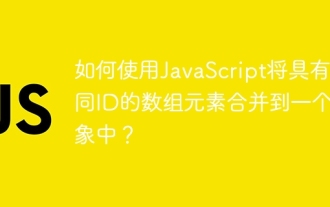
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
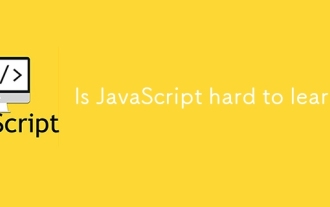
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
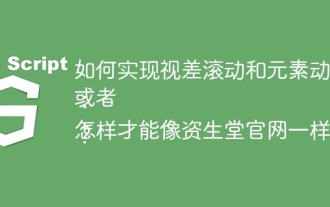
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
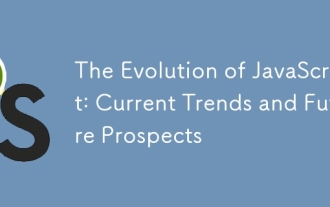
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
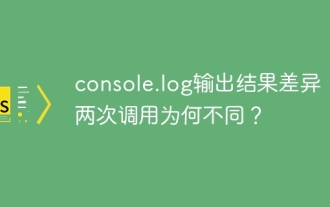
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
