


How to Initialize Non-Default Constructible std::array Elegantly with Template Metaprogramming?
Initializing Non-Default Constructible std::array Elegantly
When working with non-default constructible types in C , initializing an std::array can be challenging, especially when the array size is determined by a template parameter.
To address this, we introduce a sophisticated approach that leverages sequence-type and generator concepts:
Sequence-Type seq
We define a sequence-type called seq using a template metafunction, which represents a sequence of sequential integers.
<code class="cpp">template<int ... N> struct seq { using type = seq<N...>; static const std::size_t size = sizeof ... (N); template<int I> struct push_back : seq<N..., I> {}; };</code>
Generator genseq
Using this sequence, we construct a generator genseq that generates sequences of increasing length:
<code class="cpp">template<int N> struct genseq : genseq<N-1>::type::template push_back<N-1> {}; template<> struct genseq<0> : seq<> {}; template<int N> using genseq_t = typename genseq<N>::type;</code>
repeat() Function
With the sequence in place, we define a repeat() function to create arrays initialized with the same value:
<code class="cpp">template<typename T, int...N> auto repeat(T value, seq<N...>) -> std::array<T, sizeof...(N)> { //unpack N, repeating `value` sizeof...(N) times //note that (X, value) evaluates to value return {(N, value)...}; }</code>
Usage
To initialize an std::array of non-default constructible type, we simply call repeat() with the desired value and the sequence generated by genseq_t
<code class="cpp">template<typename T, int N> void f(T value) { //genseq_t<N> is seq<0,1,...N-1> std::array<T, N>> items = repeat(value, genseq_t<N>{}); }</code>
This solution ensures elegant and efficient initialization of std::array, effectively eliminating the need for cumbersome manual repetition when n is a large template parameter.
The above is the detailed content of How to Initialize Non-Default Constructible std::array Elegantly with Template Metaprogramming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


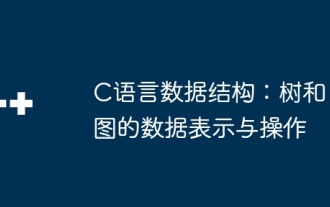
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
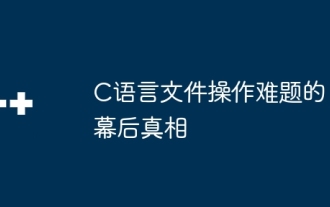
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
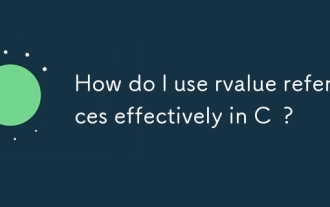
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
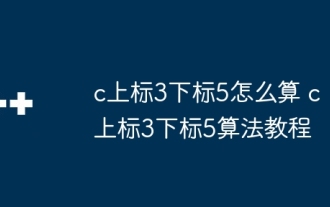
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
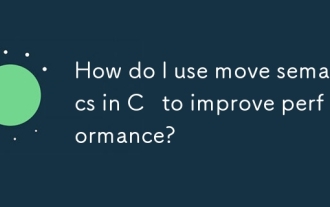
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
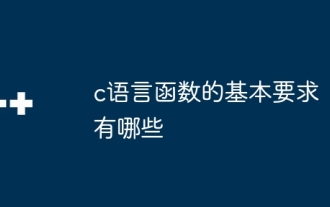
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
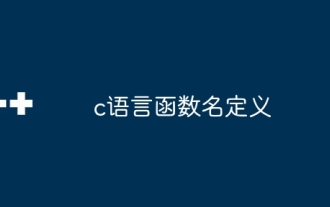
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
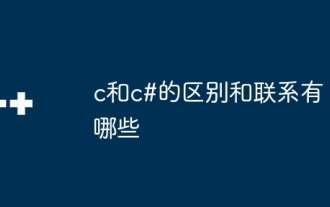
Although C and C# have similarities, they are completely different: C is a process-oriented, manual memory management, and platform-dependent language used for system programming; C# is an object-oriented, garbage collection, and platform-independent language used for desktop, web application and game development.
