


How to Unit Test GraphQL Resolvers in Go with Testify and gqlgen/client?
Unit Testing GraphQL in Go using testify
In GraphQL schema, the Query struct defines operations that trigger the retrieval of data and the Mutation struct defines operations that modify the state. When testing you'll need to mock the objects that perform the actual CRUD operations and assert that the expected calls are made.
For the following example, we'll be using the testify and gqlgen/client packages:
graph/schema.graphql
type Query { user(loginname: String!): UserDetail } type Mutation { validateAccessToken(accesstoken: String!): UserEntity } type User { loginname: String avatarUrl: String } type UserEntity { id: ID! loginname: String avatarUrl: String } type UserDetail { loginname: String avatarUrl: String githubUsername: String createAt: String score: Int }
graph/resolver/resolver.go
<code class="go">package resolver import ( "github.com/mrdulin/gqlgen-cnode/services" ) type Resolver struct { UserService services.UserService }</code>
services/user.go
<code class="go">package services type UserService interface { GetUserByLoginname(loginname string) *model.UserDetail ValidateAccessToken(accesstoken string) *model.UserEntity }</code>
graph/resolver/root.resolver.go
<code class="go">package resolver import ( "context" "github.com/mrdulin/gqlgen-cnode/graph/generated" "github.com/mrdulin/gqlgen-cnode/graph/model" ) func (r *mutationResolver) ValidateAccessToken(ctx context.Context, accesstoken string) (*model.UserEntity, error) { return r.UserService.ValidateAccessToken(accesstoken), nil } func (r *queryResolver) User(ctx context.Context, loginname string) (*model.UserDetail, error) { return r.UserService.GetUserByLoginname(loginname), nil } // Mutation returns generated.MutationResolver implementation. func (r *Resolver) Mutation() generated.MutationResolver { return &mutationResolver{r} } // Query returns generated.QueryResolver implementation. func (r *Resolver) Query() generated.QueryResolver { return &queryResolver{r} } type mutationResolver struct{ *Resolver } type queryResolver struct{ *Resolver }</code>
To simulate the GraphQL requests and responses, we can create mock objects for the services:
mocks/userService.go
<code class="go">package mocks import ( "github.com/mrdulin/gqlgen-cnode/graph/model" "github.com/stretchr/testify/mock" ) type MockedUserService struct { mock.Mock } func (s *MockedUserService) GetUserByLoginname(loginname string) *model.UserDetail { args := s.Called(loginname) return args.Get(0).(*model.UserDetail) } func (s *MockedUserService) ValidateAccessToken(accesstoken string) *model.UserEntity { args := s.Called(accesstoken) return args.Get(0).(*model.UserEntity) }</code>
Finally, in our test file, we can import these mocks and use them to test our GraphQL resolvers:
graph/resolver/root.resolver_test.go
<code class="go">package resolver_test import ( "testing" "github.com/99designs/gqlgen/client" "github.com/99designs/gqlgen/graphql/handler" "github.com/mrdulin/gqlgen-cnode/graph/generated" "github.com/mrdulin/gqlgen-cnode/graph/model" "github.com/mrdulin/gqlgen-cnode/graph/resolver" "github.com/mrdulin/gqlgen-cnode/mocks" "github.com/stretchr/testify/mock" "github.com/stretchr/testify/require" ) var ( loginname = "mrdulin" avatarURL = "avatar.jpg" score = 50 createAt = "1900-01-01" ) func TestMutationResolver_ValidateAccessToken(t *testing.T) { t.Run("should validate accesstoken correctly", func(t *testing.T) { testUserService := new(mocks.MockedUserService) resolvers := resolver.Resolver{UserService: testUserService} c := client.New(handler.NewDefaultServer(generated.NewExecutableSchema(generated.Config{Resolvers: &resolvers}))) ue := model.UserEntity{ID: "123", User: model.User{Loginname: &loginname, AvatarURL: &avatarURL}} testUserService.On("ValidateAccessToken", mock.AnythingOfType("string")).Return(&ue) var resp struct { ValidateAccessToken struct{ ID, Loginname, AvatarUrl string } } q := ` mutation { validateAccessToken(accesstoken: "abc") { id, loginname, avatarUrl } } ` c.MustPost(q, &resp) testUserService.AssertExpectations(t) require.Equal(t, "123", resp.ValidateAccessToken.ID) require.Equal(t, "mrdulin", resp.ValidateAccessToken.Loginname) require.Equal(t, "avatar.jpg", resp.ValidateAccessToken.AvatarUrl) }) } func TestQueryResolver_User(t *testing.T) { t.Run("should query user correctly", func(t *testing.T) { testUserService := new(mocks.MockedUserService) resolvers := resolver.Resolver{UserService: testUserService} c := client.New(handler.NewDefaultServer(generated.NewExecutableSchema(generated.Config{Resolvers: &resolvers}))) u := model.UserDetail{User: model.User{Loginname: &loginname, AvatarURL: &avatarURL}, Score: &score, CreateAt: &createAt} testUserService.On("GetUserByLoginname", mock.AnythingOfType("string")).Return(&u) var resp struct { User struct { Loginname, AvatarURL, CreateAt string Score int } } q := ` query GetUser($loginname: String!) { user(loginname: $loginname) { loginname avatarUrl createAt score } } ` c.MustPost(q, &resp, client.Var("loginname", "mrdulin")) testUserService.AssertCalled(t, "GetUserByLoginname", "mrdulin") require.Equal(t, "mrdulin", resp.User.Loginname) require.Equal(t, "avatar.jpg", resp.User.AvatarURL) require.Equal(t, 50, resp.User.Score) require.Equal(t, "1900-01-01", resp.User.CreateAt) }) }</code>
This test will make sure that the correct calls are made to the service methods, and that the responses are formatted as expected. The test coverage report should show full coverage of the resolver functions.
The above is the detailed content of How to Unit Test GraphQL Resolvers in Go with Testify and gqlgen/client?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










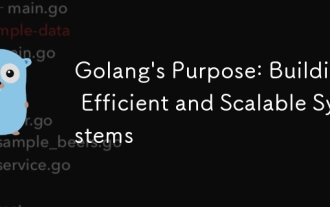
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
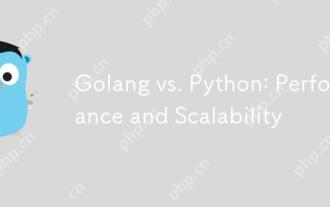
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
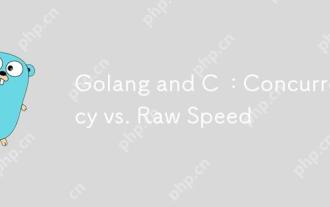
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
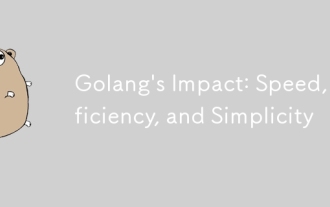
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
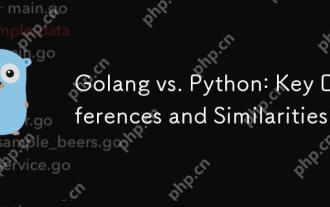
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
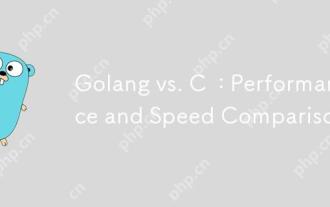
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
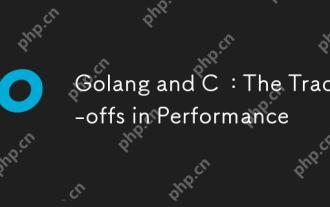
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
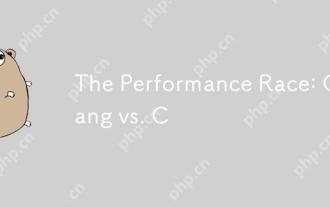
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
