


How to Read Data until CRLF Delimiter in Golang using bufio.Scanner?
How to Read Multiline Data Until CRLF Delimiter in Golang with bufio
In Golang's bufio package, the ReadBytes, ReadSlice, and ReadString functions are commonly used for reading data delimited by specific bytes or strings. However, none of these functions provide an easy way to read data until a CRLF delimiter, as required in Beanstalkd's protocol.
Using bufio.Scanner
A better approach is to use the bufio.Scanner type to create a custom split function that looks for CRLF delimiters. This allows you to read data until a CRLF delimiter is encountered. Here's how to do it:
<code class="go">import ( "bufio" "bytes" ) func ScanCRLF(data []byte, atEOF bool) (advance int, token []byte, err error) { if atEOF && len(data) == 0 { return 0, nil, nil } if i := bytes.Index(data, []byte{'\r', '\n'}); i >= 0 { // We have a full newline-terminated line. return i + 2, dropCR(data[0:i]), nil } // If we're at EOF, we have a final, non-terminated line. Return it. if atEOF { return len(data), dropCR(data), nil } // Request more data. return 0, nil, nil } func dropCR(data []byte) []byte { if len(data) > 0 && data[len(data)-1] == '\r' { return data[0 : len(data)-1] } return data }</code>
Using Your Custom Scanner
Now, you can wrap your io.Reader with your custom scanner and use it to read data until CRLF delimiters:
<code class="go">reader := bufio.NewReader(conn) scanner := bufio.NewScanner(reader) scanner.Split(ScanCRLF) for scanner.Scan() { fmt.Println(scanner.Text()) } if err := scanner.Err(); err != nil { fmt.Printf("Invalid input: %s", err) }</code>
Alternative Approach: Reading a Specific Number of Bytes
You can also read a specific number of bytes if you know the expected number of bytes in the data. However, this approach is less robust and requires careful validation to ensure that you're not reading too much or too little data. To read a specific number of bytes using the bufio.Reader type:
<code class="go">nr_of_bytes := read_number_of_butes_somehow(conn) buf := make([]byte, nr_of_bytes) reader.Read(buf)</code>
Handling Error Conditions
Both the above approaches require careful handling of error conditions, especially when dealing with partial reads and premature EOFs. Ensure that you validate the input and handle errors as appropriate.
The above is the detailed content of How to Read Data until CRLF Delimiter in Golang using bufio.Scanner?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










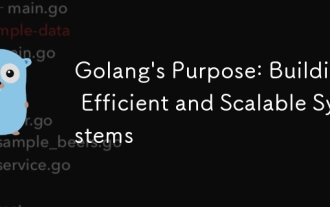
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
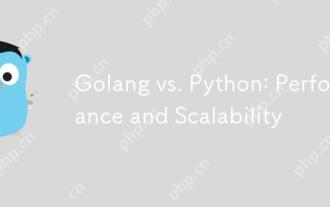
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
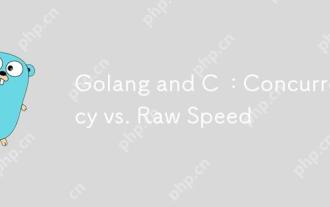
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
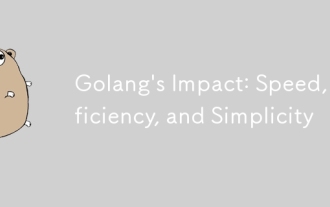
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
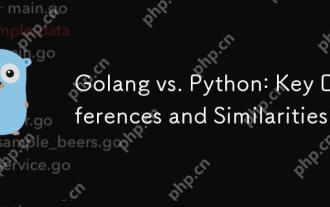
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
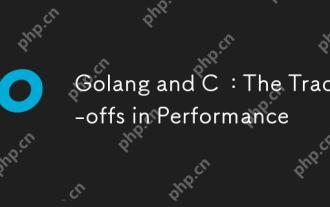
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
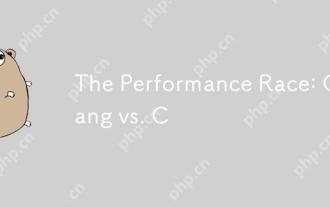
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
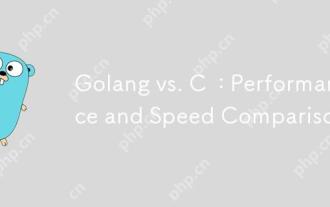
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
