How to Implement a Thread-Safe LRU Cache in Java Without External Libraries?
A Comprehensive Guide to Implementing LRU Cache in Java
In the realm of software development, efficiently managing cache capabilities often proves crucial. LRU (Least Recently Used) cache, specifically, stands out as a widely employed algorithm for optimizing memory utilization and accessing recently used data. This article delves into the intricacies of implementing an LRU cache in Java without relying on external libraries.
Data Structures for Multithreaded Environments
When implementing an LRU cache in a multithreaded environment, it becomes imperative to consider appropriate data structures that can effectively handle concurrency. One viable approach involves utilizing the combination of LinkedHashMap and Collections#synchronizedMap. LinkedHashMap provides the desired functionality for maintaining FIFO order, while Collections#synchronizedMap ensures thread-safe access.
Alternative Concurrent Collections
Java offers a plethora of concurrent collections that could potentially serve as alternatives in LRU cache implementation. ConcurrentHashMap, for instance, is designed for highly concurrent scenarios and exhibits efficient lock-free operations. However, it does not inherently retain insertion order.
Extending ConcurrentHashMap
One promising approach involves extending ConcurrentHashMap and incorporating the logic utilized by LinkedHashMap to preserve insertion order. By leveraging the capabilities of both data structures, it is possible to achieve a highly concurrent LRU cache.
Implementation Details
Here's the gist of the aforementioned implementation strategy:
<code class="java">private class LruCache<A, B> extends LinkedHashMap<A, B> { private final int maxEntries; public LruCache(final int maxEntries) { super(maxEntries + 1, 1.0f, true); this.maxEntries = maxEntries; } @Override protected boolean removeEldestEntry(final Map.Entry<A, B> eldest) { return super.size() > maxEntries; } } Map<String, String> example = Collections.synchronizedMap(new LruCache<String, String>(CACHE_SIZE));</code>
This implementation combines the FIFO ordering capabilities of LinkedHashMap with the thread safety of Collections#synchronizedMap.
Conclusion
Implementing an LRU cache in Java presents a valuable opportunity for developers to explore various data structures and concurrency concepts. The optimal approach depends on the specific performance requirements and constraints of the application at hand. By leveraging the available options, it is possible to design and implement an efficient LRU cache that effectively improves memory utilization and data access patterns.
The above is the detailed content of How to Implement a Thread-Safe LRU Cache in Java Without External Libraries?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










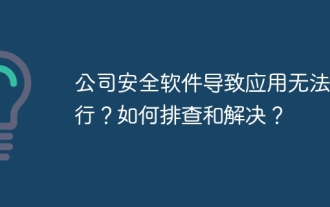
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
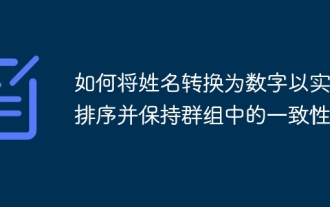
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
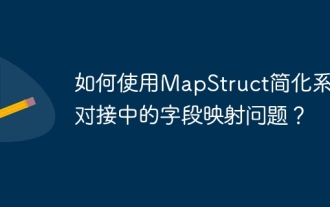
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
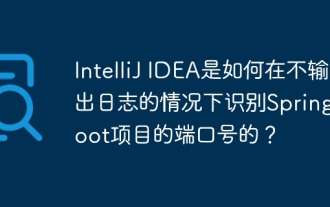
Start Spring using IntelliJIDEAUltimate version...
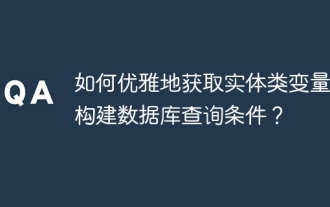
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
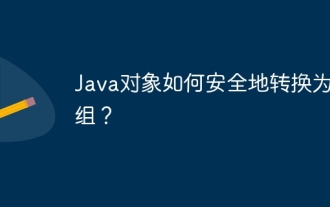
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
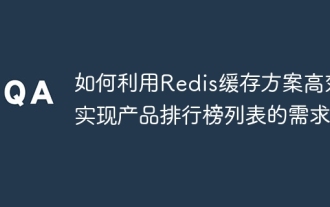
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
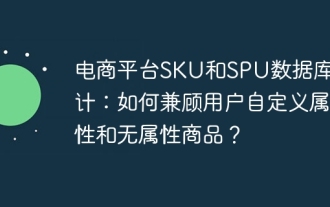
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
