How to Unpack a Slice of Slices into a Variadic Function in Go?
Variadic Function and Unpacked Slice of Slices
When working with a variadic function that accepts multiple arguments, handling a slice of slices can present a challenge. Here's a specific instance where unpacking a slice of slices can cause a compiler error:
Consider the following variadic function:
<code class="go">func unpack(args ...interface{})</code>
If we have a slice of interfaces, we can pass it directly to the function without any issues:
<code class="go">slice := []interface{}{1, 2, 3} unpack(slice) // works unpack(slice...) // also works</code>
However, if we have a slice of slices of interfaces, attempting to pass an unpacked version results in a compilation error:
<code class="go">sliceOfSlices := [][]interface{}{ []interface{}{1, 2}, []interface{}{101, 102}, } unpack(sliceOfSlices) // works unpack(sliceOfSlices...) // compiler error</code>
The error message is:
cannot use sliceOfSlices (type [][]interface {}) as type []interface {} in argument to unpack
To understand this behavior, we need to delve into the specification for passing arguments to variadic parameters in Go:
"If the final argument is assignable to a slice type []T, it may be passed unchanged as the value for a ...T parameter if the argument is followed by ...."
In this case, the variadic parameter is ...interface{}, and a direct assignment of sliceOfSlices (which is of type [][]interface{}) to args (of type []interface{}) is not valid.
So, how can we send the unpacked contents of sliceOfSlices to unpack()? The solution lies in creating a new slice of type []interface{}, copying the elements, and then passing it using ....
<code class="go">var sliceOfSlices2 []interface{} for _, v := range sliceOfSlices { sliceOfSlices2 = append(sliceOfSlices2, v) } unpack(sliceOfSlices2...)</code>
This method ensures that each inner slice is represented as an element in a single slice, making it compatible with the ...interface{} variadic parameter.
The above is the detailed content of How to Unpack a Slice of Slices into a Variadic Function in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










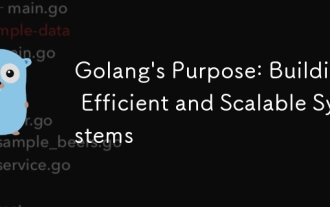
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
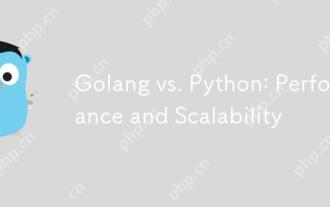
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
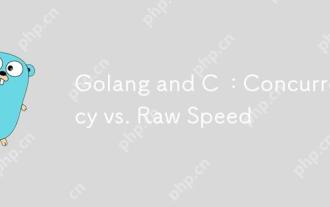
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
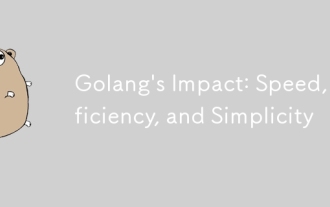
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
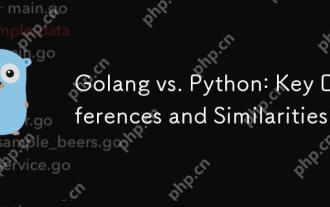
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
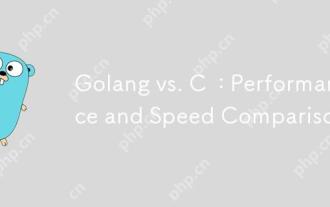
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
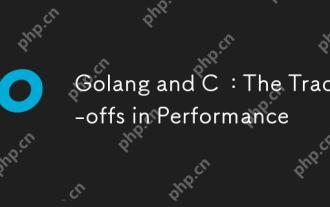
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
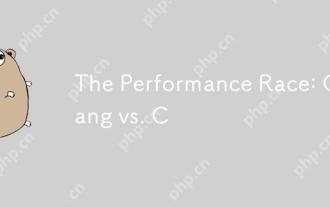
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
