


How Can I Calculate Rolling Standard Deviation on a 1D NumPy Array Using a Rolling Window?
Rolling Window Implementation for 1D Arrays in NumPy
For efficient handling of rolling windows on 1D arrays, NumPy provides a useful implementation. Let's consider a scenario where we have a 1D NumPy array called observations. To calculate the rolling standard deviations with a window length of n, we can leverage the following approach:
<code class="python">import numpy as np n = 5 # Example window length # Create a rolling window for the observations rolling_window = np.lib.stride_tricks.as_strided(observations, shape=(len(observations) - n + 1, n), strides=(observations.strides[0],)) # Apply the standard deviation function to each window rolling_stdev = np.std(rolling_window, axis=1)</code>
This code snippet efficiently applies the NumPy std function to each window, resulting in the desired rolling standard deviation values. Note that you can replace np.std with any other function you wish to apply to the windowed data.
The above is the detailed content of How Can I Calculate Rolling Standard Deviation on a 1D NumPy Array Using a Rolling Window?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




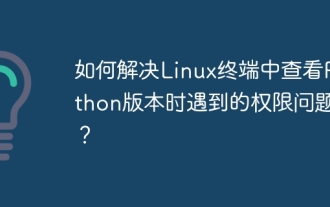
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
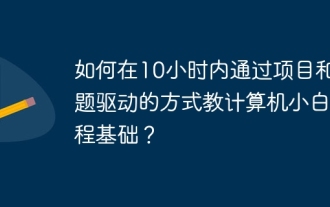
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
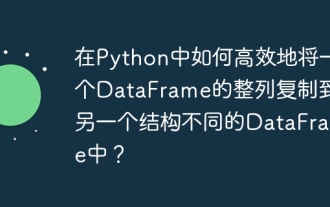
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
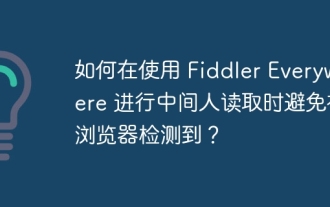
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
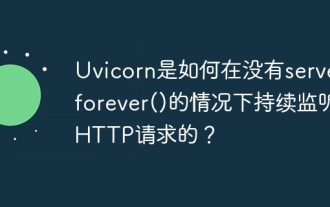
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
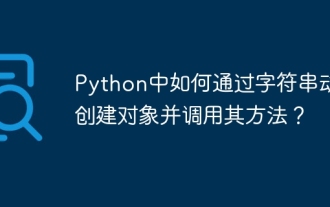
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
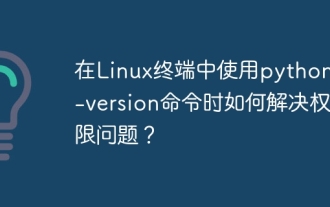
Using python in Linux terminal...
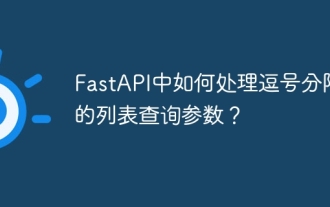
Fastapi ...
