


How does the \'new\' operator in JavaScript work internally, and what are the key steps involved in object creation using this operator?
Understanding the Inner Workings of the "new" Operator in JavaScript
Considered one of the enigmatic aspects of JavaScript, the "new" operator has fascinated developers for its role in object creation. This article delves into the "new" operator's intricate mechanism, revealing its essence through an alternative implementation.
The Function Behind the "new" Operator
The "new" operator internally invokes the [[Construct]] method of the function that follows it. This method plays a pivotal role in the object creation process:
-
Object Initialization:
- It initializes a pristine, native JavaScript object.
-
Prototype Linkage:
- It establishes a connection between the newly created object's internal [[Prototype]] property and the function's prototype property.
- If the function's prototype property is not an object, it opts for Object.prototype instead.
-
Function Invocation:
- The function associated with the "new" operator is executed, utilizing the newly created object as its "this" value.
The Return Value Enigma
The outcome of the "new" operator hinges on the return value of the invoked function:
- Primitive Return Value: If the function returns a primitive value (e.g., Number, String), the internally created object is returned.
- Object Return Value: If the function returns an object, the internal object is discarded, and the returned object takes precedence.
An Alternative Implementation
To comprehend the inner workings of the "new" operator, let's explore an alternative implementation that mimics its behavior:
<code class="javascript">function NEW(f) { var obj, ret, proto; proto = Object(f.prototype) === f.prototype ? f.prototype : Object.prototype; obj = Object.create(proto); ret = f.apply(obj, Array.prototype.slice.call(arguments, 1)); if (Object(ret) === ret) { return ret; } return obj; }</code>
Example Usage:
<code class="javascript">function Foo (arg) { this.prop = arg; } Foo.prototype.inherited = 'baz'; var obj = NEW(Foo, 'bar'); console.log(obj.prop); // 'bar' console.log(obj.inherited); // 'baz' console.log(obj instanceof Foo); // true</code>
Conclusion
Through this alternative implementation, we gained a comprehensive understanding of the "new" operator's vital role in object creation and prototype chain establishment. Its intricate mechanism, when understood, enables developers to harness the power of JavaScript's object-oriented programming capabilities.
The above is the detailed content of How does the \'new\' operator in JavaScript work internally, and what are the key steps involved in object creation using this operator?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
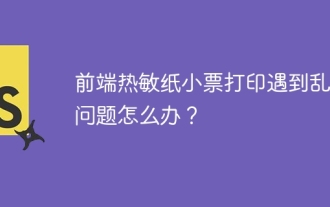
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
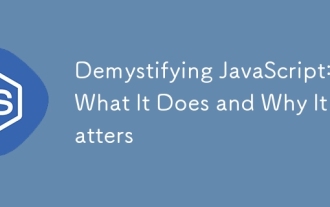
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
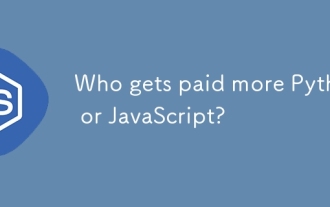
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
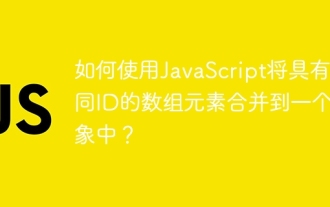
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
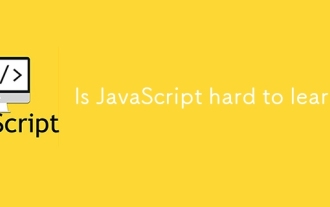
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
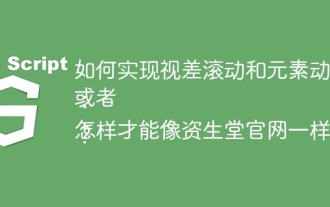
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
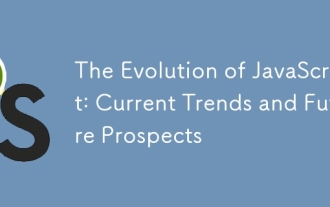
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
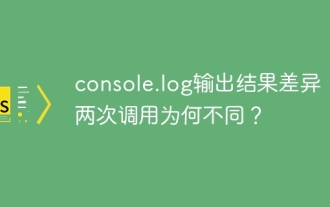
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
