


How to Transform a 2D Array into a 3D Array by Grouping Elements Based on a Column Value?
Oct 28, 2024 pm 08:05 PMGrouping 2D Array Data Based on Column Value to Create a 3D Array
In programming, you may encounter the need to organize data in multidimensional arrays. To efficiently manage complex data, it becomes essential to group elements according to specific criteria. This article tackles the specific challenge of grouping a 2D array by the values in a specific column, transforming it into a 3D array for enhanced visualization and manipulation.
Consider the following multidimensional array containing customer information:
<code class="php">$data = [ ['cust' => 'XT8900', 'type' => 'standard', 'level' => 1], ['cust' => 'XT8944', 'type' => 'standard', 'level' => 1], ['cust' => 'XT8922', 'type' => 'premier', 'level' => 3], ['cust' => 'XT8816', 'type' => 'premier', 'level' => 3], ['cust' => 'XT7434', 'type' => 'standard', 'level' => 7], ];</code>
The objective is to group these customer records based on the 'level' column and create a 3D array where each level contains an array of customer information. The desired result should look something like this:
<code class="php">$result = [ 1 => [ ['cust' => 'XT8900', 'type' => 'standard'], ['cust' => 'XT8944', 'type' => 'standard'], ], 3 => [ ['cust' => 'XT8922', 'type' => 'premier'], ['cust' => 'XT8816', 'type' => 'premier'], ], 7 => [ ['cust' => 'XT7434', 'type' => 'standard'], ], ];</code>
There are several approaches to achieve this but let's explore an effective solution:
-
Sorting the Data:
Begin by sorting the 2D array by the 'level' column. This can be done using the usort() function to compare the 'level' values of each element. -
Grouping the Sorted Data:
Now, iterate through the sorted data and create a temporary array. For each entry, retrieve the 'level' value as the key and store the entire entry as the value. This will effectively group the customer records by level.<code class="php">foreach ($data as $key => &$entry) { $level_arr[$entry['level']][$key] = $entry; }</code>
Copy after login
The resulting $level_arr will have the desired structure, with each level containing an array of customer information.
-
Converting to a 3D Array:
Now, convert the temporary $level_arr into the target 3D array. This involves setting the keys of the 3D array to the level values and the values to the corresponding customer arrays from $level_arr.<code class="php">$result = []; foreach ($level_arr as $level => $customer_data) { $result[$level] = array_values($customer_data); }</code>
Copy after login -
Final Result:
The $result variable is now the 3D array with customer records grouped by the 'level' column.
By following these steps, you can effectively group data in a 2D array using a specific column value and construct a 3D array for enhanced data manipulation and visualization. This approach offers flexibility and efficiency when dealing with complex data structures.
The above is the detailed content of How to Transform a 2D Array into a 3D Array by Grouping Elements Based on a Column Value?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
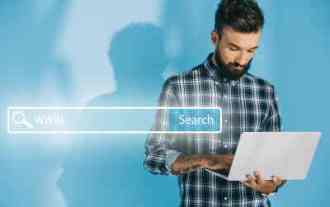
11 Best PHP URL Shortener Scripts (Free and Premium)
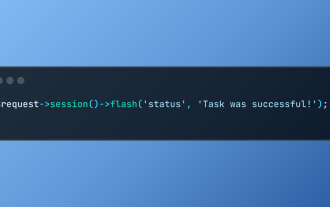
Working with Flash Session Data in Laravel
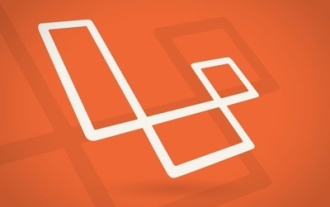
Build a React App With a Laravel Back End: Part 2, React
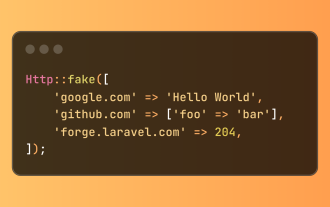
Simplified HTTP Response Mocking in Laravel Tests
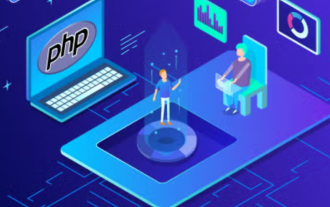
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
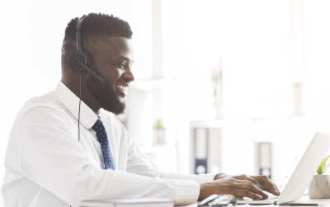
12 Best PHP Chat Scripts on CodeCanyon
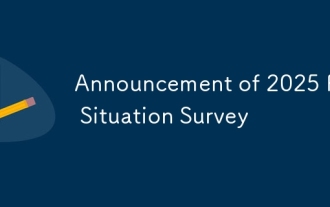
Announcement of 2025 PHP Situation Survey
