How to Select a Random Product from a Firebase Database in Node.js?
How to retrieve a unique random product from a Firebase database in Node.js:
In this context, we aim to retrieve a single, random product from a node named "products" in a Firebase database. Here are two approaches:
Classic Approach:
This involves retrieving all product records from the "products" node and selecting a random one:
const rootRef = firebase.database().ref(); const productsRef = rootRef.child("products"); // Listen for a single snapshot of the products node productsRef.once('value').then((snapshot) => { // Get all product names const productNames = []; snapshot.forEach((child) => { productNames.push(child.val().name); }); // Select a random product name const randomProductName = productNames[Math.floor(Math.random() * productNames.length)]; // Get the specific product data using the random name rootRef.child(`products/${randomProductName}`).once('value').then((product) => { console.log(product.val()); }); });
Denormalized Approach:
In this technique, we create a separate node called "productIds" that contains only the IDs of all products. This allows us to retrieve a random product ID without fetching all product records:
const rootRef = firebase.database().ref(); const productIdsRef = rootRef.child("productIds"); // Listen for a single snapshot of the productIds node productIdsRef.once('value').then((snapshot) => { // Get all product IDs const productIds = Object.keys(snapshot.val()); // Select a random product ID const randomProductId = productIds[Math.floor(Math.random() * productIds.length)]; // Get the specific product data using the random ID rootRef.child(`products/${randomProductId}`).once('value').then((product) => { console.log(product.val()); }); });
The above is the detailed content of How to Select a Random Product from a Firebase Database in Node.js?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










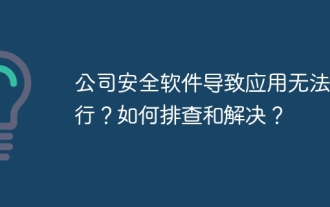
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
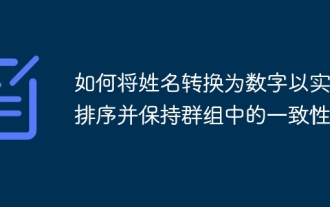
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
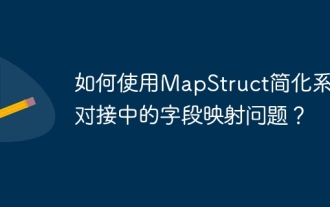
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
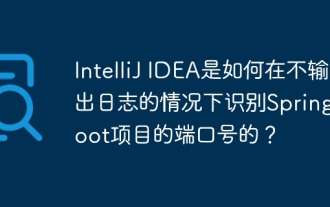
Start Spring using IntelliJIDEAUltimate version...
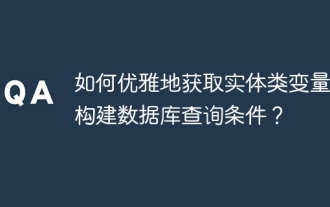
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
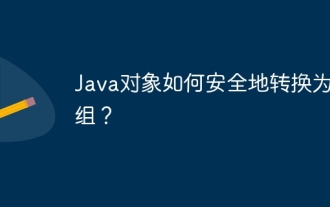
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
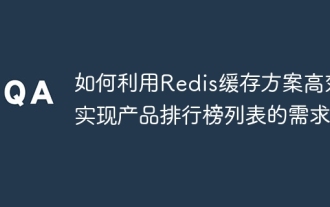
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
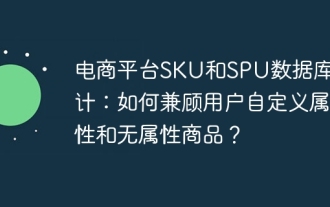
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
