


How to Simplify Form Validation in PHP: A Concise Function for Checking Empty Posted Variables
PHP: Simplified Function for Checking Empty Posted Variables
Form validation is crucial to prevent malicious input and ensure complete data. While it's common to manually check each posted variable, the task becomes tedious and error-prone as the form complexity increases.
To streamline this process, we explore a simpler approach that eliminates the need for lengthy conditional statements.
Simplified Function
The following function provides a more concise and efficient solution:
<code class="php">// Required field names $required = array('login', 'password', 'confirm', 'name', 'phone', 'email'); // Loop over field names, check existence and emptiness $error = false; foreach($required as $field) { if (empty($_POST[$field])) { $error = true; } } if ($error) { echo "All fields are required."; } else { echo "Proceed..."; }</code>
This function eliminates the repetitive syntax for each variable and instead utilizes a loop to iterate over the required field names. It simplifies the code, making it more readable and maintainable.
Implementation
To implement this function, simply replace the original conditional statements with the simplified function:
<code class="php">if (isset($_POST['Submit'])) { $required = array('login', 'password', 'confirm', 'name', 'phone', 'email'); $error = false; foreach($required as $field) { if (empty($_POST[$field])) { $error = true; } } if ($error) { echo "All fields are required."; } else { echo "Proceed..."; } }</code>
Advantages
Using this simplified function provides several advantages:
- Conciseness: It significantly reduces the amount of code required for form validation.
- Readability: It makes the validation code clearer and easier to understand.
- Maintainability: It improves the future maintenance and expansion of the form validation process.
The above is the detailed content of How to Simplify Form Validation in PHP: A Concise Function for Checking Empty Posted Variables. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
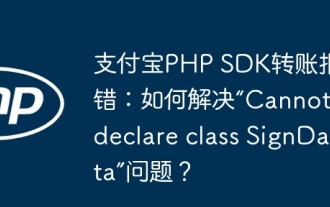
Alipay PHP...
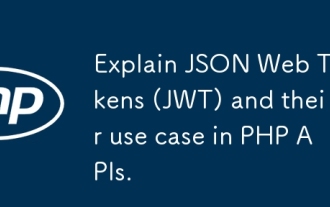
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
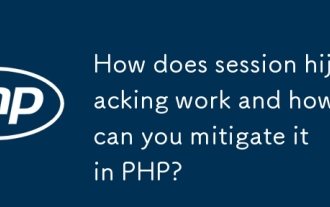
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
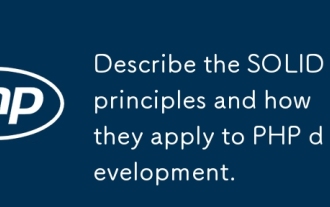
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
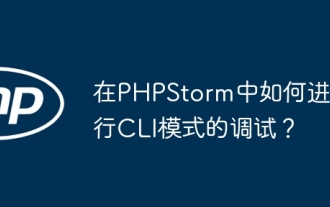
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
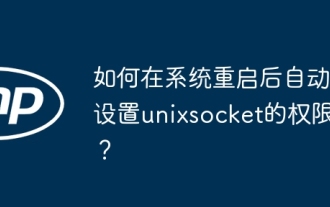
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
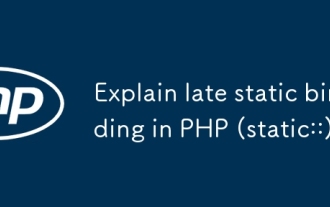
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
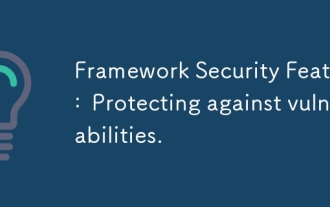
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
