


How can I use Go reflection to select functions based on their input and output types?
Selecting Functions by Type in Go
Consider a scenario where you have an array or slice of functions of varying types. To process these functions efficiently, you may want to select only the ones that return or receive specific types of data, such as integers. This can be achieved using the Go reflection package.
The reflection package provides the means to introspect the type of a value at runtime. By inspecting the input and output types of a function, you can filter out the ones that meet your criteria.
Consider the following example program:
<code class="go">package main import ( "fmt" "reflect" ) func main() { funcs := make([]interface{}, 3, 3) // I use interface{} to allow any kind of func funcs[0] = func(a int) int { return a + 1 } // good funcs[1] = func(a string) int { return len(a) } // good funcs[2] = func(a string) string { return ":(" } // bad for _, fi := range funcs { f := reflect.ValueOf(fi) functype := f.Type() good := false for i := 0; i < functype.NumIn(); i++ { if "int" == functype.In(i).String() { good = true // yes, there is an int among inputs break } } for i := 0; i < functype.NumOut(); i++ { if "int" == functype.Out(i).String() { good = true // yes, there is an int among outputs break } } if good { fmt.Println(f) } } }</code>
In this program, we create a slice of functions ([]interface{}) to store functions of varying types. We then iterate through this slice and for each function, we use reflection to obtain its type (reflect.ValueOf(fi).Type()). By inspecting the input and output types, we can determine whether the function meets our criteria. In this case, we check for functions that receive or return integers. If such a function is found, it is printed to the output.
The reflection package provides a powerful mechanism for introspecting Go values, including functions. By leveraging this capability, you can filter out functions based on their types at runtime, allowing for efficient and flexible code operations.
The above is the detailed content of How can I use Go reflection to select functions based on their input and output types?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










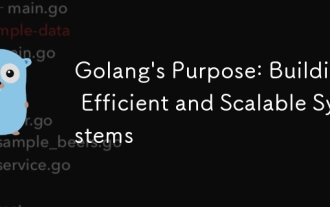
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
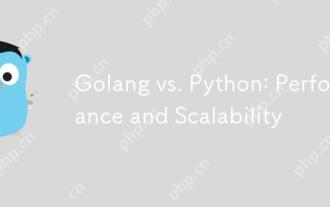
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
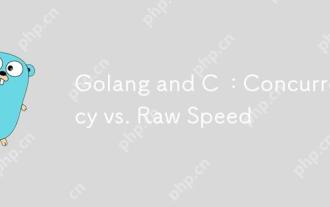
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
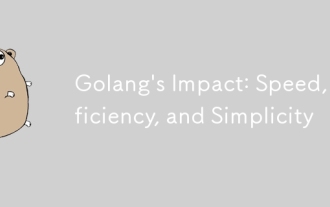
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
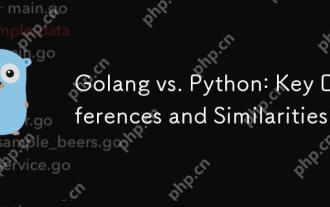
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
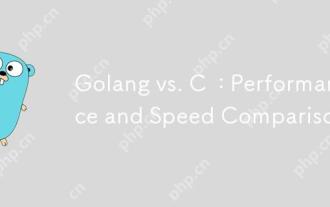
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
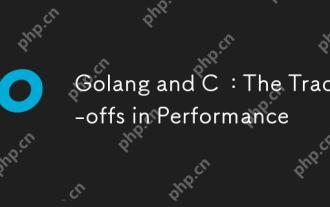
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
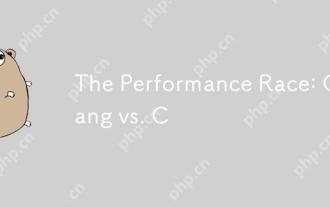
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
