


How to Properly Close HTTP Requests and Handle Background Processing in Go?
Closing HTTP Requests and Background Processing in Go
In Go's HTTP handling, it's essential to properly close requests to ensure resource management and prevent potential errors. When responding to an HTTP request with a 202 Accepted status code and continuing processing in the background, several approaches are available.
Using Return or WriteHeader with Goroutines
In the code snippet provided, two methods are presented:
<code class="go">// Return: func index(w http.ResponseWriter, r *http.Request) { w.WriteHeader(http.StatusAccepted) go sleep() return } // WriteHeader with goroutine: func index(w http.ResponseWriter, r *http.Request) { w.WriteHeader(http.StatusAccepted) go sleep() }</code>
Proper Closing with Return
When returning from an HTTP handler function, it signals that the request is complete. This means that the ResponseWriter and RequestBody should not be used or read from after returning.
In the first approach, by returning after the WriteHeader call and launching the sleep goroutine, the request is closed and processing continues in the background. This is the recommended approach as it adheres to Go's handling guidelines.
Omission of Return
The final return statement is unnecessary as Go automatically returns from the function once its last statement is executed. However, returning explicitly can enhance code readability.
Automatic 200 OK Response
If no HTTP headers are set when returning from the handler, Go will automatically set the response to 200 OK. Thus, for a 202 Accepted response, you must explicitly set the WriteHeader as demonstrated in the example.
Precautions for Goroutine Usage
When using goroutines within handlers, it's crucial to avoid accessing the ResponseWriter and Request values after returning. These values may be reused, and attempting to read them can lead to unpredictable behavior.
Conclusion
Properly closing HTTP requests in Go is achieved by returning from the handler. This ensures resource cleanup and prevents potential errors. When continuing processing in the background, launch goroutines before returning. Adopting these guidelines ensures efficient HTTP handling practices in Go applications.
The above is the detailed content of How to Properly Close HTTP Requests and Handle Background Processing in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










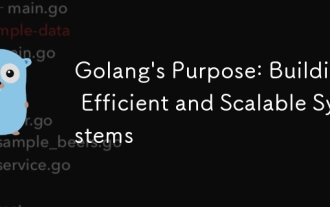
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
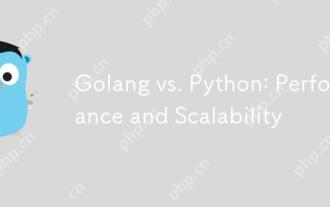
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
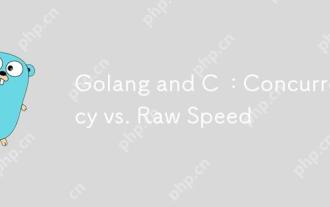
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
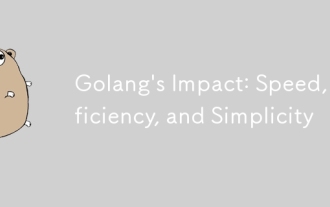
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
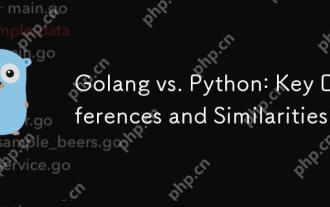
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
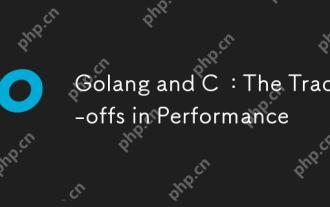
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
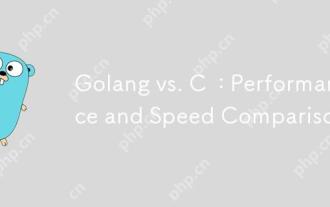
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
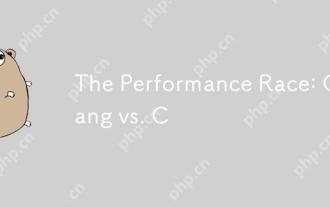
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
