


How to Efficiently Select Random Products from a Large Firebase Database in Node?
Getting Unique Random Products in Node Firebase
To retrieve a single random product from a node database over 1000 records, consider two approaches: the classic solution and a denormalized approach.
Classic Solution
<br>ListView listView = (ListView) findViewById(R.id.list_view);<br>ArrayAdapter arrayAdapter = new ArrayAdapter<>(context, android.R.layout.simple_list_item_1, randomProductList);<br>listView.setAdapter(arrayAdapter);<br>DatabaseReference rootRef = FirebaseDatabase.getInstance().getReference();<br>DatabaseReference productsRef = rootRef.child("products"); //Added call to .child("products")<br>ValueEventListener valueEventListener = new ValueEventListener() {</p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">@Override public void onDataChange(DataSnapshot dataSnapshot) { List<String> productList = new ArrayList<>(); for(DataSnapshot ds : dataSnapshot.getChildren()) { String name = ds.child("name").getValue(String.class); productList.add(name); } int productListSize = productList.size(); List<String> randomProductList = new ArrayList<>(); randomProductList.add(new Random().nextInt(productListSize)); //Add the random product to list arrayAdapter.notifyDatasetChanged(); } @Override public void onCancelled(DatabaseError databaseError) { Log.d(TAG, "Error: ", task.getException()); //Don't ignore errors! }
};
productsRef.addListenerForSingleValueEvent(valueEventListener);
This approach loops through all product nodes, adds their names to a list, and randomly selects one.
Denormalized Approach
Create a separate "productIds" node to avoid downloading large amounts of data:
<br>Firebase-root<br> |<br> --- products<br> | |<br> | --- productIdOne<br> | | |<br> | | --- //details<br> | |<br> | --- productIdTwo<br> | |<br> | --- //details<br> | <br> --- productIds</p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false"> | --- productIdOne: true | --- productIdTwo: true | --- //And so on
<br>DatabaseReference rootRef = FirebaseDatabase.getInstance().getReference();<br>DatabaseReference productIdsRef = rootRef.child("productIds");<br>ValueEventListener valueEventListener = new ValueEventListener() {</p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">@Override public void onDataChange(DataSnapshot dataSnapshot) { List<String> productIdsList = new ArrayList<>(); for(DataSnapshot ds : dataSnapshot.getChildren()) { String productId = ds.getKey(); productIdsList.add(productId); } int productListSize = productList.size(); List<String> randomProductList = new ArrayList<>(); DatabaseReference productIdRef = rootRef.child("products").child(productIdsList.get(new Random().nextInt(int productListSize)); ValueEventListener eventListener = new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { String name = dataSnapshot.child("name").getValue(String.class); Log.d("TAG", name); } @Override public void onCancelled(DatabaseError databaseError) { Log.d(TAG, "Error: ", task.getException()); //Don't ignore errors! } }; productIdRef.addListenerForSingleValueEvent(eventListener); } @Override public void onCancelled(DatabaseError databaseError) { Log.d(TAG, "Error: ", task.getException()); //Don't ignore errors! }
};
productIdsRef.addListenerForSingleValueEvent(valueEventListener);
This approach queries the "productIds" node to get a random product ID, then queries the "products" node for specific details.
The above is the detailed content of How to Efficiently Select Random Products from a Large Firebase Database in Node?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










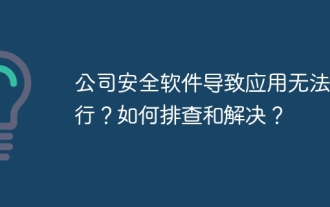
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
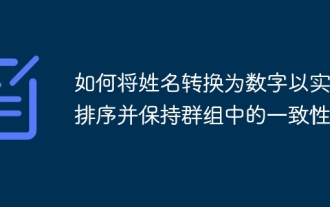
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
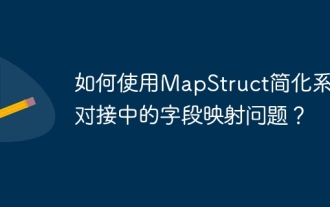
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
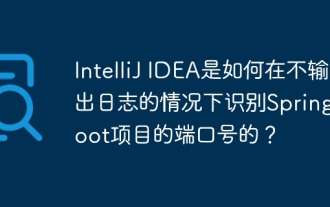
Start Spring using IntelliJIDEAUltimate version...
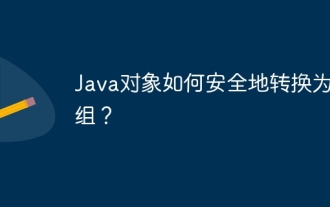
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
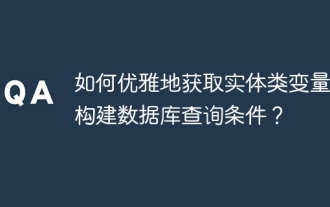
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
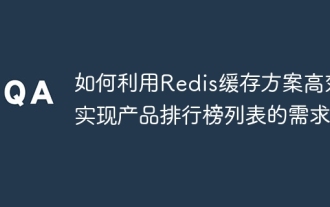
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
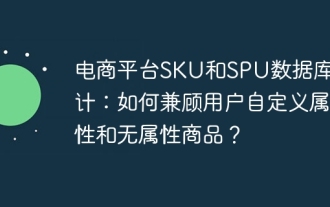
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
