


How can I generate a Bash script using Go templates with switch and ForEach to iterate through dependencies and output corresponding echo messages based on their type?
Golang Template with Switch & ForEach
This code demonstration focuses on utilizing a template in Go to produce a bash script. The script requires ForEach iteration through dependencies, identifying their types, and outputting corresponding echo messages. A switch statement is implemented to handle the type of each dependency.
package main import ( "log" "text/template" "gopkg.in/yaml.v2" "os" ) type File struct { TypeVersion string `yaml:"_type-version"` Dependency []Dependency } type Dependency struct { Name string Type string CWD string Install []Install } type Install map[string]string var data = ` _type-version: "1.0.0" dependency: - name: ui type: runner cwd: /ui install: - name: api - name: ui2 type: runner2 cwd: /ui2 install: - name: api2 ` func main() { f := File{} err := yaml.Unmarshal([]byte(data), &f) if err != nil { log.Fatalf("error: %v", err) } const t = ` #!/bin/bash {{range .Dependency}} echo "type is {{.Type}}" echo "cwd is {{.CWD}}" {{range .Install}} echo "install {{.name}}" {{end}} {{end}} ` tt := template.Must(template.New("").Parse(t)) err = tt.Execute(os.Stdout, f) if err != nil { log.Println("executing template:", err) } }
The provided data is Unmarshalled into a File structure, which is then ready for template execution. This modified code generates a script with:
#!/bin/bash echo "type is runner" echo "cwd is /ui" echo "install api" echo "type is runner2" echo "cwd is /ui2" echo "install api2"
The above is the detailed content of How can I generate a Bash script using Go templates with switch and ForEach to iterate through dependencies and output corresponding echo messages based on their type?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










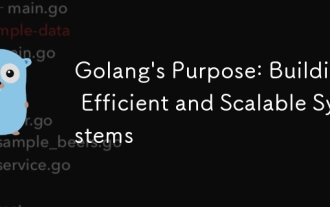
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
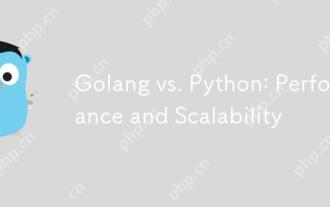
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
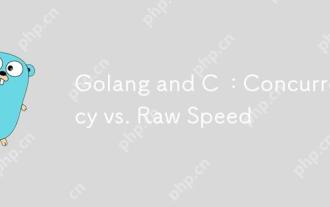
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
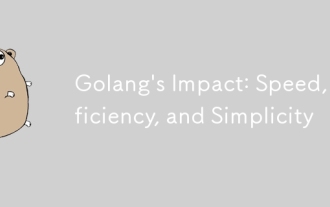
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
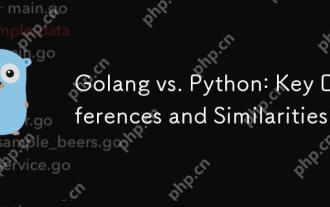
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
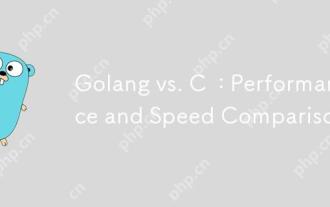
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
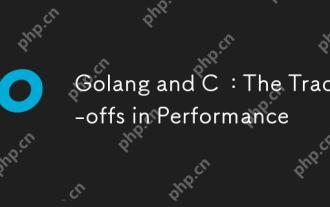
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
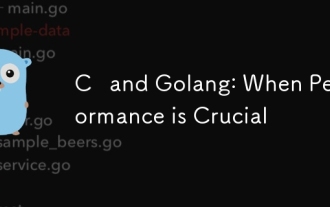
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
