


Why Do Lambda Expressions Capture the Final Value of Iterator Variables in C#?
Oct 29, 2024 am 11:02 AMUsing Lambda Expressions with Iterators in C#
In C#, lambda expressions provide a concise and convenient way to define anonymous functions. However, a common pitfall when using lambda expressions with iterators is that the captured variable is not the current value of the iterator variable, but rather the final value.
Consider the following code:
<code class="c#">Type[] types = new Type[] { typeof(string), typeof(float), typeof(int) }; List<PrintHelloType> helloMethods = new List<PrintHelloType>(); foreach (var type in types) { var sayHello = new PrintHelloType(greeting => SayGreetingToType(type, greeting)); helloMethods.Add(sayHello); } foreach (var helloMethod in helloMethods) { Console.WriteLine(helloMethod("Hi")); }</code>
When you execute this code, you might expect it to print the following output:
Hi String Hi Single Hi Int32
However, the actual output is:
Hi Int32 Hi Int32 Hi Int32
This is because the lambda expression captures the loop variable type, not its value at the time the lambda is defined. Therefore, when the lambda is executed, it uses the final value of type, which is Int32.
To fix this issue and obtain the desired output, you can use a local variable to capture the current value of the iterator variable, as shown in the following code:
<code class="c#">foreach (var type in types) { var newType = type; var sayHello = new PrintHelloType(greeting => SayGreetingToType(newType, greeting)); helloMethods.Add(sayHello); }</code>
In this case, the lambda expression captures the local variable newType, which is assigned the current value of type. This ensures that the lambda expression uses the correct value of type when it is executed.
The above is the detailed content of Why Do Lambda Expressions Capture the Final Value of Iterator Variables in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
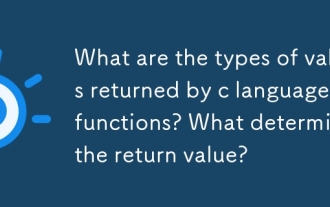
What are the types of values returned by c language functions? What determines the return value?
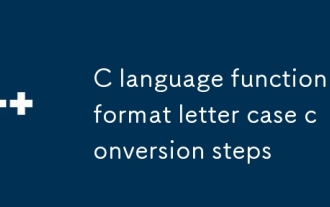
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
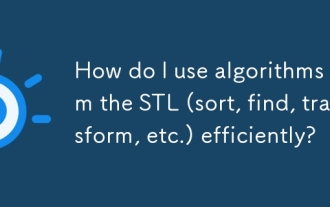
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
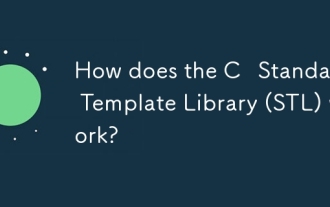
How does the C Standard Template Library (STL) work?
