


How do you Check if a Property Exists in a Javascript Object with a Dynamic Property Name?
Determining Object Property Existence Using a Dynamic Property Name
When dealing with objects in JavaScript, it's often necessary to check if a specific property exists. However, if the property name is stored in a variable, conventional methods may not work effectively.
In the given code snippet, the developer attempts to check if the myObj object has a property named 'prop', but the myProp variable is incorrectly defined with a string concatenation. As a result, the code searches for a non-existent property 'myProp'.
To address this issue, several alternative approaches can be used:
1. Using hasOwnProperty()
The hasOwnProperty() method verifies if the specified property is directly defined in the object (not inherited from its prototype).
<code class="js">var myProp = 'prop'; if(myObj.hasOwnProperty(myProp)){ alert("yes, i have that property"); }</code>
2. Using the in Operator
The in operator checks if a given property exists in an object, regardless of whether it's directly defined or inherited.
<code class="js">var myProp = 'prop'; if(myProp in myObj){ alert("yes, i have that property"); }</code>
3. Direct Property Name Check
If the property name is known with certainty, it can be checked directly without the need for a variable.
<code class="js">if('prop' in myObj){ alert("yes, i have that property"); }</code>
Note: The hasOwnProperty() method ignores inherited properties, while the in operator includes them. Therefore, the choice of approach depends on whether inherited properties are relevant to the check being performed.
The above is the detailed content of How do you Check if a Property Exists in a Javascript Object with a Dynamic Property Name?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
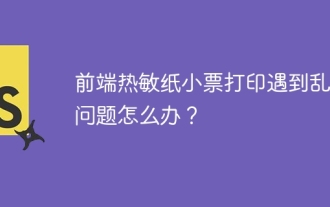
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
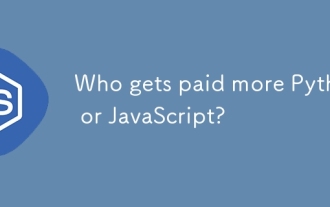
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
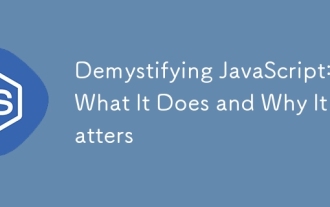
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
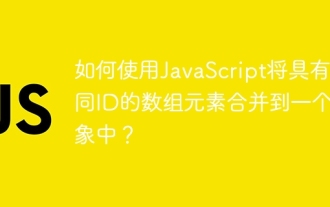
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
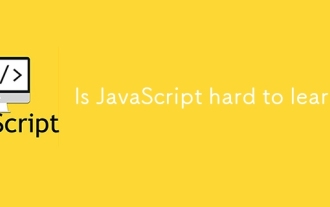
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
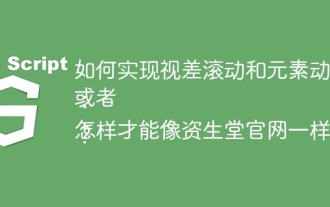
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
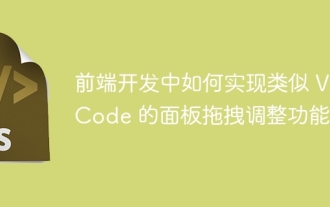
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
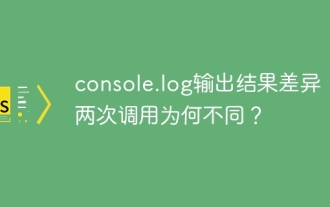
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
