How Can I Acquire Locks with Deadlines in Go?
Acquiring Locks with Deadlines in Go
In Go, the sync.Mutex provides exclusive access to a shared resource. However, it lacks the ability to acquire locks with deadlines, like TryLock or LockBefore. This presents challenges in certain scenarios, such as latency-sensitive services or updating objects within a time limit.
Solution: Using a Channel as a Mutex
An alternative to sync.Mutex is to utilize a channel with a buffer size of one as a mutex. This approach provides a simple and effective way to implement lock acquisition with deadlines.
Lock:
<code class="go">l := make(chan struct{}, 1) l <- struct{}{} // Acquire the lock</code>
Unlock:
<code class="go"><-l // Release the lock</code>
Try Lock:
<code class="go">select { case l <- struct{}{}: // Lock acquired <-l default: // Lock not acquired }</code>
Try Lock with Timeout:
<code class="go">select { case l <- struct{}{}: // Lock acquired <-l case <-time.After(time.Minute): // Lock not acquired }</code>
By using a channel as a mutex, you can achieve the desired behavior of attempting to acquire a lock within a specified deadline. This method provides a flexible and efficient solution for scenarios where lock acquisition needs to be time-bounded.
The above is the detailed content of How Can I Acquire Locks with Deadlines in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
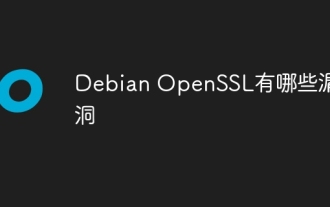
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
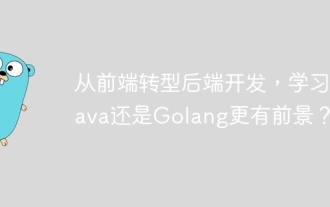
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
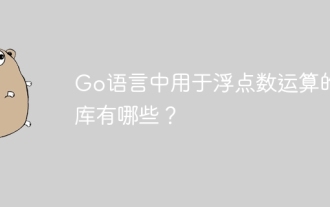
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
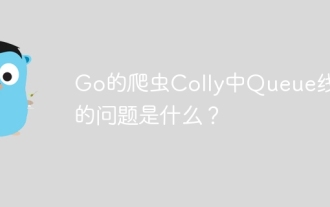
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
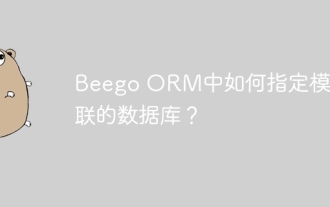
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
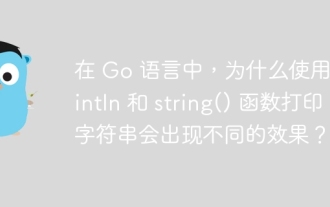
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
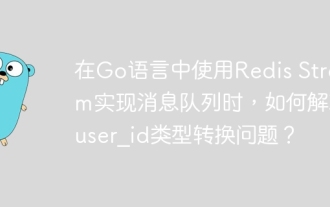
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
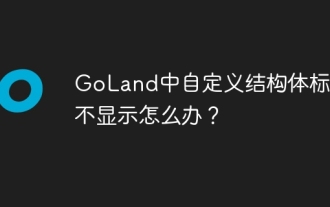
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
