How to Access a Database Connection from a Function in Go?
Referencing an Open Database Connection within a Function in Go
When working with databases in Go, it's common to establish a connection to the database in the main function and then utilize this connection throughout the application. However, what happens when you want to encapsulate database operations within a function?
Consider the following scenario. In your main function, you open a database connection:
<code class="go">func main() { db, err := sql.Open("sqlite3", "./house.db") checkErr(err) ... }</code>
Now, you're interested in creating a function that adds rows to the database based on a passed struct:
<code class="go">func addRow(row Room) error { stmt, err := db.Prepare("INSERT INTO Rooms (Name, Size, WindowCount, WallDecorationType, Floor) VALUES(?, ?, ?, ?, ?)") _, err = stmt.Exec(row.Name , row.Size , row.WindowCount , row.WallDecorationType , row.Floor) return err }</code>
But here lies the challenge: the addRow() function has no knowledge of the db variable defined in the main function.
How to Handle Database Connection References
Depending on the nature of your application, you have several options to reference the database connection within the addRow() function:
- Global Variable: Declare db as a global variable. This means it will be accessible anywhere in your program, including the addRow() function. Note that using global variables should be used sparingly.
- Function Parameter: Pass the db connection as a parameter to the addRow() function. This provides more explicit control over the connection, and it allows you to use different database connections if needed.
<code class="go">func addRow(db *sql.DB, row Room) error</code>
- Method: Create a struct that manages the database connection and make addRow() a method on that struct. This approach encapsulates the database connection within the struct.
<code class="go">type dbConn struct { db *sql.DB } func (conn dbConn) addRow(row Room) error</code>
Example Usage
Let's demonstrate the usage of the global variable approach:
<code class="go">var db *sql.DB func main() { db, err := sql.Open("sqlite3", "./house.db") checkErr(err) // create room Room{} err = addRow(room) checkErr(err) }</code>
By declaring db as a global variable, it becomes accessible to the addRow() function anywhere in the program.
Keep in mind that the approach you choose should be tailored to the specific requirements of your application, its architecture, and performance considerations.
The above is the detailed content of How to Access a Database Connection from a Function in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










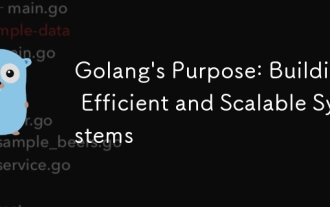
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
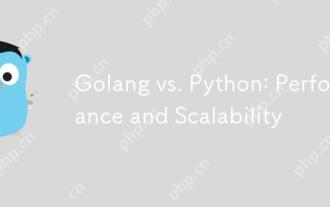
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
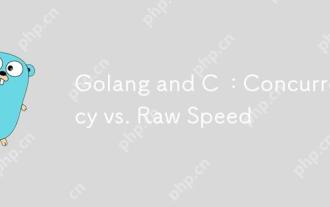
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
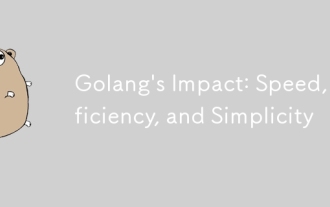
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
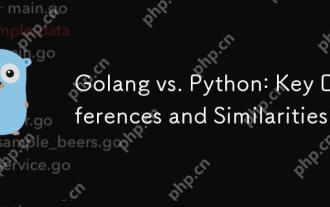
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
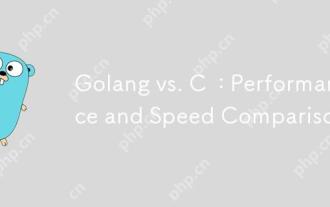
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
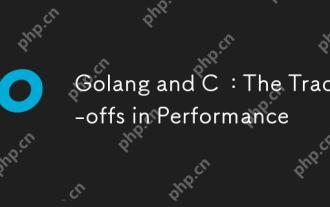
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
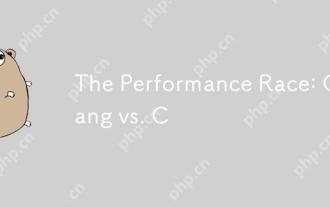
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
