


How can I create a broadcast receiver in Android to detect and handle both internet connectivity changes and sudden internet loss?
Managing Internet Connectivity Changes in Android: A Comprehensive Guide
In Android, detecting connectivity changes is crucial for applications that require reliable internet access. However, relying solely on connectivity changes can leave web-based apps vulnerable to sudden internet loss. This article explores a comprehensive solution to create a broadcast receiver that listens for internet connectivity changes.
Original Problem:
The provided code monitors connectivity changes but fails to detect sudden internet loss without changes in connectivity.
Solution:
To address this issue, we need to create a broadcast receiver that specifically listens for internet connectivity changes, disconnected from connectivity changes. Here's how:
Implementing the Broadcast Receiver:
Create a new class, NetworkChangeReceiver, that extends BroadcastReceiver. In this class, override the onReceive method to handle internet connectivity changes:
<code class="java">public class NetworkChangeReceiver extends BroadcastReceiver { @Override public void onReceive(final Context context, final Intent intent) { // Check the connectivity status int status = NetworkUtil.getConnectivityStatusString(context); Log.e("Network reciever status", String.valueOf(status)); // Perform actions based on connectivity status if (status == NetworkUtil.NETWORK_STATUS_NOT_CONNECTED) { // Handle no internet connectivity new ForceExitPause(context).execute(); } else { // Handle internet connectivity restored new ResumeForceExitPause(context).execute(); } } }</code>
Creating Utility Methods:
Include a util class, NetworkUtil, to abstract the logic for determining connectivity status:
<code class="java">public class NetworkUtil { // Define connectivity types and status public static final int TYPE_WIFI = 1; public static final int TYPE_MOBILE = 2; public static final int TYPE_NOT_CONNECTED = 0; public static final int NETWORK_STATUS_NOT_CONNECTED = 0; public static final int NETWORK_STATUS_WIFI = 1; public static final int NETWORK_STATUS_MOBILE = 2; // Get connectivity status as an integer public static int getConnectivityStatus(Context context) { // Obtain the ConnectivityManager ConnectivityManager cm = (ConnectivityManager) context.getSystemService(Context.CONNECTIVITY_SERVICE); // Get the active network NetworkInfo activeNetwork = cm.getActiveNetworkInfo(); // Check if there is an active network if (activeNetwork != null) { // Check network type if (activeNetwork.getType() == ConnectivityManager.TYPE_WIFI) { return TYPE_WIFI; } else if (activeNetwork.getType() == ConnectivityManager.TYPE_MOBILE) { return TYPE_MOBILE; } } // No active network, return no connectivity return TYPE_NOT_CONNECTED; } // Get connectivity status as a string (Wifi, Mobile, Not Connected) public static int getConnectivityStatusString(Context context) { int conn = getConnectivityStatus(context); int status = 0; // Map connectivity status to string switch (conn) { case TYPE_WIFI: status = NETWORK_STATUS_WIFI; break; case TYPE_MOBILE: status = NETWORK_STATUS_MOBILE; break; case TYPE_NOT_CONNECTED: status = NETWORK_STATUS_NOT_CONNECTED; break; } return status; } }</code>
Updating the Manifest File:
Add the necessary permissions and declare the broadcast receiver in your AndroidManifest.xml file:
<code class="xml"><uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-permission android:name="android.permission.INTERNET" /> <receiver android:name="NetworkChangeReceiver" android:label="NetworkChangeReceiver" > <intent-filter> <action android:name="android.net.conn.CONNECTIVITY_CHANGE" /> <action android:name="android.net.wifi.WIFI_STATE_CHANGED" /> </intent-filter> </receiver></code>
With this comprehensive solution, your Android application can now monitor internet connectivity changes and handle them accordingly, ensuring a more reliable user experience.
The above is the detailed content of How can I create a broadcast receiver in Android to detect and handle both internet connectivity changes and sudden internet loss?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










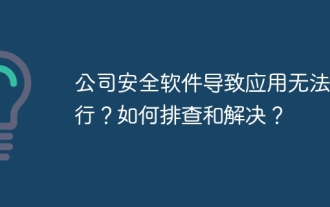
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
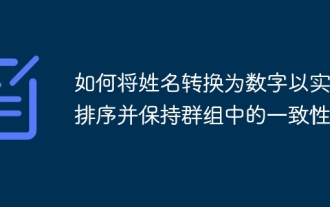
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
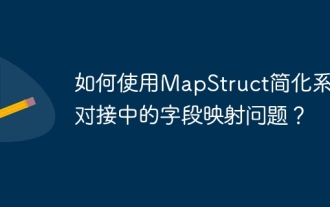
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
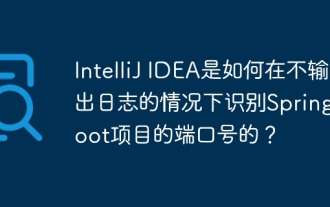
Start Spring using IntelliJIDEAUltimate version...
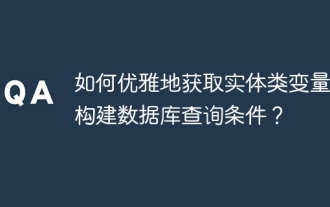
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
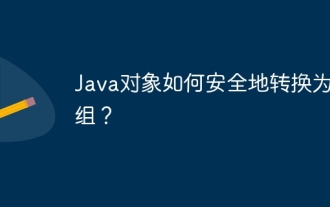
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
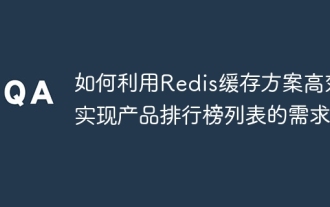
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
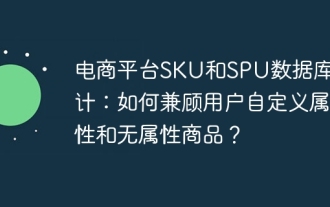
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
