How to Implement Delimited I/O Functions in C for Protocol Buffers?
C Equivalents for Protocol Buffers Delimited I/O Functions in Java
In C , there are no direct equivalents to the "Delimited" I/O functions introduced in Java's Protocol Buffers API version 2.1.0. These functions allow for reading and writing multiple Protocol Buffers messages with length prefixes attached.
Wire Format of Java Delimited I/O Functions
The Java "Delimited" I/O functions use a wire format that is not documented by Google. However, the author of the C and Java protobuf libraries has provided unofficial implementations of similar functions:
<code class="cpp">bool writeDelimitedTo( const google::protobuf::MessageLite& message, google::protobuf::io::ZeroCopyOutputStream* rawOutput) { // Write the size. const int size = message.ByteSize(); output.WriteVarint32(size); // Serialize the message. uint8_t* buffer = output.GetDirectBufferForNBytesAndAdvance(size); if (buffer != NULL) { message.SerializeWithCachedSizesToArray(buffer); } else { message.SerializeWithCachedSizes(&output); } return true; } bool readDelimitedFrom( google::protobuf::io::ZeroCopyInputStream* rawInput, google::protobuf::MessageLite* message) { // Read the size. uint32_t size; if (!input.ReadVarint32(&size)) return false; // Limit the stream to the size of the message. google::protobuf::io::CodedInputStream::Limit limit = input.PushLimit(size); // Parse the message. if (!message->MergeFromCodedStream(&input)) return false; // Verify that the entire message was consumed. if (!input.ConsumedEntireMessage()) return false; // Release the limit. input.PopLimit(limit); return true; }</code>
These implementations ensure that the 64MB size limit is applied individually to each message and not to the entire stream. Additionally, they utilize optimizations to improve performance when the message size is relatively small.
The above is the detailed content of How to Implement Delimited I/O Functions in C for Protocol Buffers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
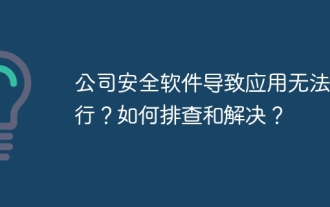
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
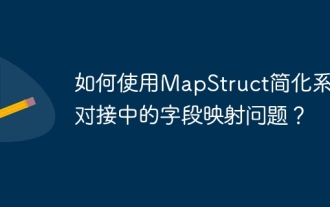
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
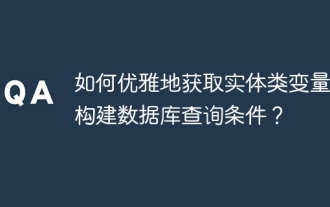
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
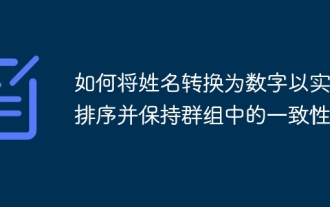
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
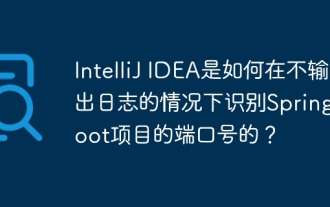
Start Spring using IntelliJIDEAUltimate version...
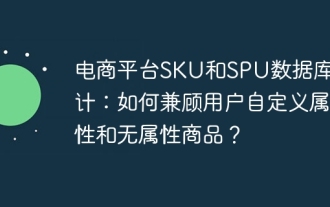
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
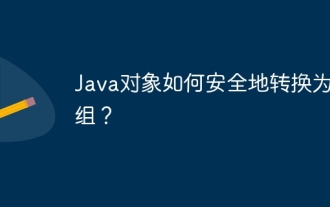
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
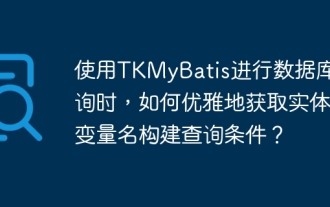
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
