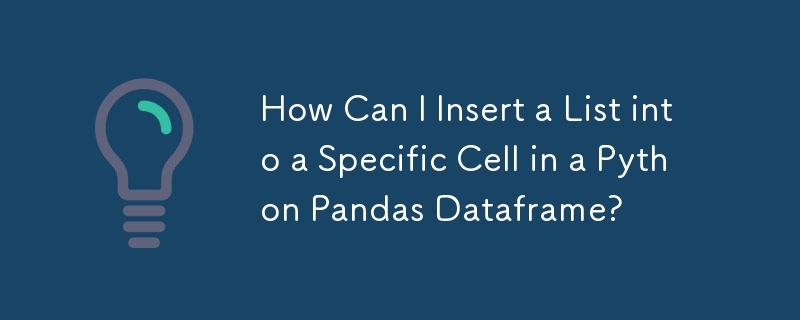
Insert List into a Cell in Python Pandas Dataframe
Inserting a list into a specific cell in a pandas dataframe can be a tricky task. Let's explore the various approaches and potential issues based on the given example:
Original Problem:
A dataframe 'df' with the following structure:
and a list 'abc' containing ['foo', 'bar']. The goal is to insert this list into cell 1B.
Efforts:
- df.ix[1,'B'] = abc: Throws a ValueError due to unequal length of keys and values.
- df.ix[1,'B'] = [abc]: Inserts a list containing the 'abc' list instead of individual elements.
- df.ix[1,'B'] = ', '.join(abc): Inserts a string instead of a list.
- df.ix[1,'B'] = [', '.join(abc)]: Inserts a one-element list containing the joined string.
Solution:
The deprecated set_value method has been replaced with at. Using at guarantees setting a single value:
1 | <code class = "python" >df.at[1, 'B' ] = [ 'foo' , 'bar' ]</code>
|
Copy after login
Additional Considerations:
- Ensure that the target column has dtype=object to accommodate list insertion.
- The same approach can be applied to insert lists into cells containing integer or string values.
- However, when inserting a list into a column containing mixed data types (integer and string), a ValueError may occur. This can be resolved by converting the column to dtype=object before insertion.
Updated Example:
Inserting the 'abc' list into df2.loc[1,'B'] and df3.loc[1,'B']:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | <code class = "python" >df2 = pd.DataFrame({
'A' : [12],
'B' : [nan],
'C' : [ 'bla' ]
})
df3 = pd.DataFrame({
'A' : [12],
'B' : [nan],
'C' : [ 'bla bla' ],
'D' : [[ 'item1' , 'item2' ], [11, 12, 13]]
})
df2.loc[1, 'B' ] = [ 'foo' , 'bar' ]
df3.loc[1, 'B' ] = [ 'foo' , 'bar' ]</code>
|
Copy after login
The above is the detailed content of How Can I Insert a List into a Specific Cell in a Python Pandas Dataframe?. For more information, please follow other related articles on the PHP Chinese website!