


Is comparing `getAbsolutePath()` and `getCanonicalPath()` a reliable way to determine if a directory in Java 1.6 is a symbolic link?
Determining Symbolic Links in Java 1.6
In a Java program operating on Unix systems, distinguishing between actual directories and symbolic links is essential. This question explores a method for identifying symbolic links to directories using a specific condition.
Question:
In the context of a DirectoryWalker class, can the following approach be used to accurately determine if a known directory instance represents a symbolic link:
<code class="java">if (file.getAbsolutePath().equals(file.getCanonicalPath())) { // real directory ---> do normal stuff } else { // possible symbolic link ---> do link stuff }</code>
Answer:
While the approach provided is a common technique to identify possible symbolic links, it cannot be considered reliable for the following reasons:
- Potential False Positives: A mismatch between the absolute and canonical paths can occur even for actual directories, such as mounted network drives.
- Platform Dependence: The behavior of file paths can vary across different operating systems and file systems.
Instead of relying on the absolute and canonical paths of the file itself, it is recommended to use the canonical path of the parent directory. This approach is more accurate in identifying symbolic links.
Here's an example from Apache Commons that implements this technique:
<code class="java">public static boolean isSymlink(File file) throws IOException { if (file == null) throw new NullPointerException("File must not be null"); File canon; if (file.getParent() == null) { canon = file; } else { File canonDir = file.getParentFile().getCanonicalFile(); canon = new File(canonDir, file.getName()); } return !canon.getCanonicalFile().equals(canon.getAbsoluteFile()); }</code>
The above is the detailed content of Is comparing `getAbsolutePath()` and `getCanonicalPath()` a reliable way to determine if a directory in Java 1.6 is a symbolic link?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
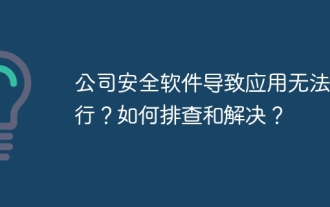
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
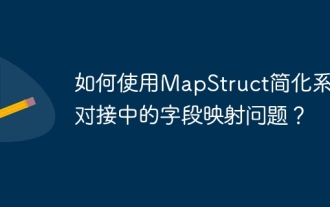
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
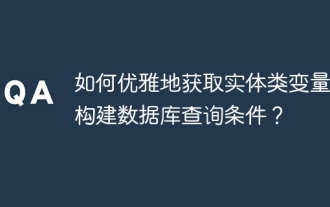
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
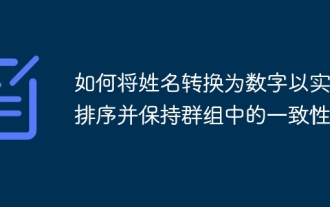
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
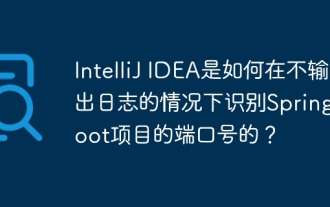
Start Spring using IntelliJIDEAUltimate version...
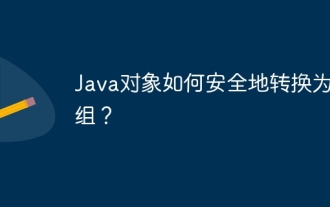
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
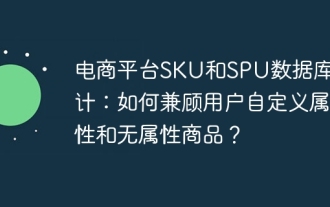
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
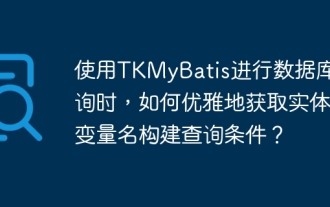
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
