How to Return Multiple Values from a Java Method?
Returning Multiple Values from a Java Method
In Java, methods typically return a single value of a specific type. However, sometimes, a method may need to return multiple values.
Problem Description
The provided code aims to return two integers, number1 and number2, from the something() method. However, the compilation fails with an error message indicating a missing return statement.
Solution
While the proposed approaches, such as creating arrays or using generic Pair classes, provide solutions to the problem, they may not be optimal in terms of type safety and readability. Instead, creating a custom class representing the desired result is a preferred approach.
Custom Class for Result
Consider creating a class named MyResult that encapsulates both integers:
<code class="java">final class MyResult { private final int first; private final int second; public MyResult(int first, int second) { this.first = first; this.second = second; } public int getFirst() { return first; } public int getSecond() { return second; } }</code>
Updated Method Signature and Implementation
Modify the something() method to return an instance of MyResult:
<code class="java">public static MyResult something() { int number1 = 1; int number2 = 2; return new MyResult(number1, number2); }</code>
Main Method Usage
In the main() method, obtain the returned values from the MyResult instance:
<code class="java">public static void main(String[] args) { MyResult result = something(); System.out.println(result.getFirst() + result.getSecond()); }</code>
This approach provides type safety and makes the program easier to understand by clearly representing the intended result.
The above is the detailed content of How to Return Multiple Values from a Java Method?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










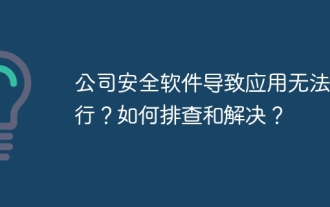
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
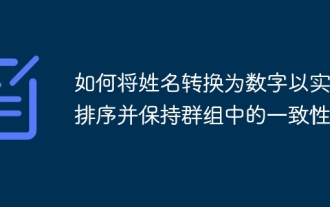
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
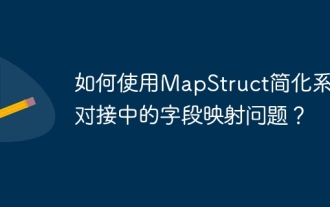
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
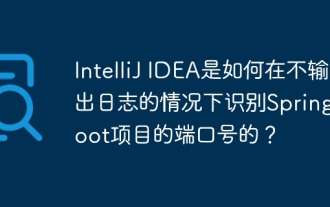
Start Spring using IntelliJIDEAUltimate version...
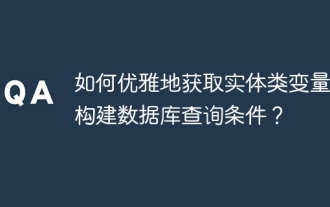
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
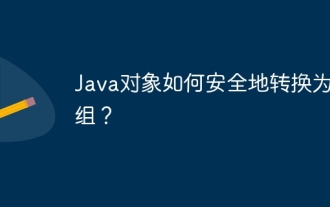
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
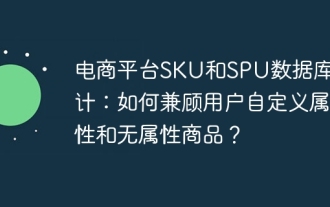
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
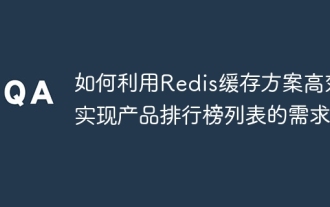
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
