


How do Regular Expression Groups in C# Capture and Access Matched Substrings?
Regular Expression Groups in C#: Understanding Match Results
Consider the following C# code block:
<code class="csharp">var pattern = @"\[(.*?)\]"; var matches = Regex.Matches(user, pattern); if (matches.Count > 0 && matches[0].Groups.Count > 1) ...</code>
This code uses a regular expression to extract bracketed text from a user input string. For the input "Josh Smith [jsmith]", the code correctly returns the following results:
matches.Count == 1 matches[0].Value == "[jsmith]"
However, the subsequent lines raise questions:
matches[0].Groups.Count == 2 matches[0].Groups[0].Value == "[jsmith]" matches[0].Groups[1].Value == "jsmith"
Match Grouping
In regular expressions, groups are used to capture specific parts of a match. By default, the entire match is captured in Group 0. Additional capturing groups can be defined using parentheses.
In the provided code, the regular expression defines a single capturing group, denoted by (.*?). This group captures the text within the square brackets (jsmith in this case). Thus:
- matches[0].Groups[0] contains the entire match, including the square brackets: [jsmith]
- matches[0].Groups[1] contains the captured text within the brackets: jsmith
Nested Groups
In more complex regular expressions, it is possible to have nested groups. In these cases, each group contains its own set of captures. However, in the given code, there is only one level of grouping, so:
- matches[0].Groups[1].Captures is always empty.
Additional Considerations
- Group 0: It is important to note that Group 0 always contains the entire match, regardless of whether any capturing groups are defined.
- Number of Groups: The number of groups in a regular expression match depends on the number of capturing groups defined in the pattern. In the provided example, there is only one capturing group, resulting in two groups total.
The above is the detailed content of How do Regular Expression Groups in C# Capture and Access Matched Substrings?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










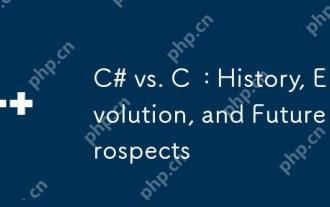
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
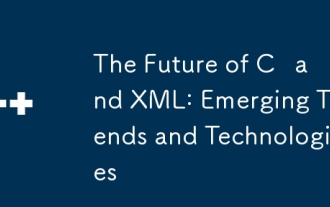
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
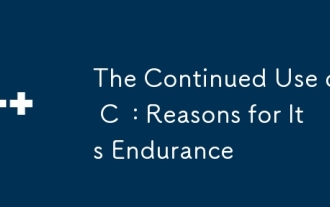
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
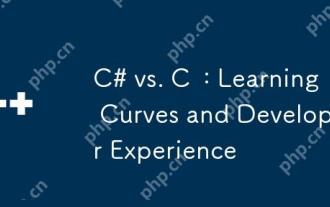
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
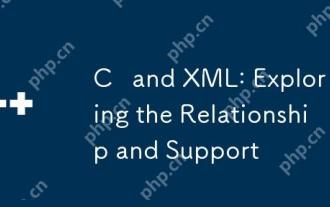
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
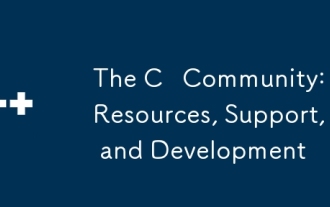
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
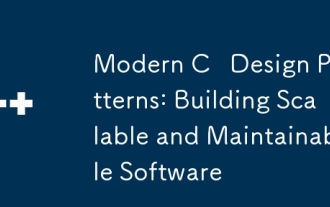
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
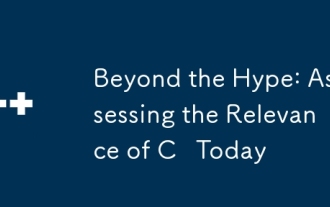
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
