How Can I Cancel Promises in JavaScript for Type-Ahead Search?
Promise - is it possible to force cancel a promise
In this scenario, the user is performing a type-ahead search, and each keystroke triggers a new backend call. As the user types faster, the older requests become irrelevant and need to be cancelled.
Promises in ES6, as they currently stand, do not support cancellation out-of-the-box. However, there is ongoing work to introduce cancellation capabilities through AbortController, a cross-platform primitive that can cancel asynchronous operations like network requests.
Using AbortController
As a modern approach, AbortController provides a signal object that can be used to cancel functions that return promises. The syntax looks like this:
<code class="javascript">async function somethingIWantToCancel({ signal } = {}) { // Use the signal object to manually adapt code for cancellation support const onAbort = (e) => { // Handle cancellation logic here }; signal.addEventListener('abort', onAbort, { once: true }); try { const response = await fetch('...', { signal }); } catch (e) { if (e.name === 'AbortError') { // Deal with cancellation in caller } else { throw e; } } finally { signal.removeEventListener('abort', onAbort); } }</code>
In this example, the somethingIWantToCancel function takes a signal parameter, which is an instance of AbortController. The signal.addEventListener listener registers an event handler for the abort event, which is triggered when the operation is cancelled. The finally block ensures that the event listener is removed when the operation is complete.
Alternatives
If AbortController is not an option, another approach is to use a third-party library like Bluebird, which provides cancellation capabilities.
Another alternative is to use a cancellation token. This involves passing a function to the method that can be called to cancel the request.
Conclusion
While ES6 promises do not have native cancellation support, AbortController and other alternatives provide ways to cancel asynchronous operations in modern JavaScript. For older scenarios, using a cancellation token or third-party library can still provide a solution.
The above is the detailed content of How Can I Cancel Promises in JavaScript for Type-Ahead Search?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
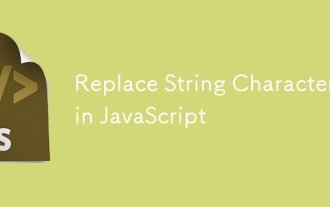
Replace String Characters in JavaScript
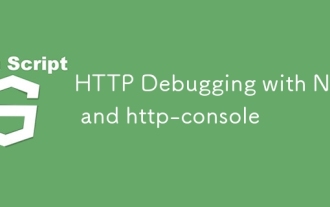
HTTP Debugging with Node and http-console
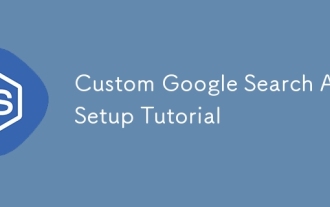
Custom Google Search API Setup Tutorial
